Sound
As of Unity 5, there aren’t any 2D/3D sounds anymore. The AudioSource now determines how sounds are treated. Go to the AudioSource component in your Inspector and change the Spatial Blend value.
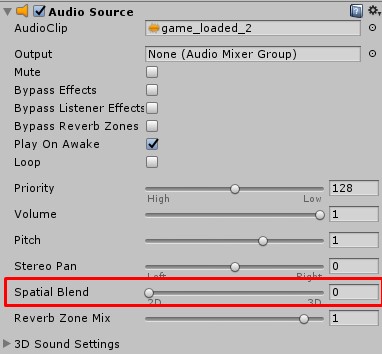
This also applies to the PlayOneShot method which instantiates a short-living game object with an AudioSource attached. In the Block Breaker game, some crack sounds could suddenly appear louder than others. To fix this, do not pass on the position of the brick but of the position of the camera. The camera has got an AudioListener attached, so all AudioSources set to 3D use the distance to determine the volume of the sound clips.
AudioSource.PlayClipAtPoint(crack, Camera.main.position, 0.8f);
If you want to use Camera.main multiple times in your script, cache the reference for reasons of performance:
private Camera cam;
void Start() {
this.cam = Camera.main;
}
void YourMethod() {
AudioSource.PlayClipAtPoint(crack, this.cam.position, 0.8f);
}
Additional information: