Hi!
Ever since I introduced the enemy in my 2D game, the player doesn’t jump anymore.
Besides, my enemy doesn’t move left or right.
If anyone can help me I would be very grateful !!
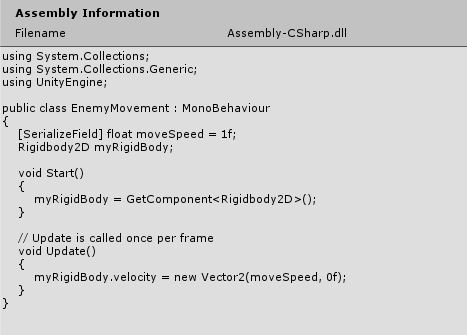
Hi!
Ever since I introduced the enemy in my 2D game, the player doesn’t jump anymore.
Besides, my enemy doesn’t move left or right.
If anyone can help me I would be very grateful !!
Hi Raluca,
Please note, it’s better to copy/paste your code and apply the code fencing characters, rather than using screenshots. Screenshots are ideal for displaying specific details from within a game engine editor or even error messages, but for code, they tend to be less readable, especially on mobile devices which can require extensive zooming and scrolling.
You also prevent those that may offer to help you the ability to copy/paste part of your code back to you with suggestions and/or corrections, meaning that they would need to type a potentially lengthy response. You will often find that people are more likely to respond to your questions if you make it as easy as possible for them to do so.
Have you already compared your code to the Lecture Project Changes which can be found in the Resources of this lecture?
Hope this helps
See also;
Here is the code for Enemy:
‘’’
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyMovement : MonoBehaviour
{
[SerializeField] float moveSpeed = 1f;
Rigidbody2D myRigidBody;
void Start()
{
myRigidBody = GetComponent<Rigidbody2D>();
}
// Update is called once per frame
void Update()
{
myRigidBody.velocity = new Vector2(moveSpeed, 0f);
}}
’ ’ ’
And the code for Player:
‘’’
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityStandardAssets.CrossPlatformInput;
public class Player : MonoBehaviour
{
//Config
[SerializeField] float runSpeed = 5f;
[SerializeField] float jumpSpeed = 5f;
[SerializeField] float climbspeed = 5f;
//State
bool isAlive = true;
//Cached component references
Rigidbody2D myRigidBody;
Animator myAnimator;
CapsuleCollider2D myBodyCollider;
BoxCollider2D myFeet;
float gravityScaleAtStart;
// Message then method
void Start()
{
myRigidBody = GetComponent<Rigidbody2D>();
myAnimator = GetComponent<Animator>();
myBodyCollider = GetComponent<CapsuleCollider2D>();
myFeet = GetComponent<BoxCollider2D>();
gravityScaleAtStart = myRigidBody.gravityScale;
}
// Update is called once per frame
void Update()
{
Run();
ClimbLadder();
FlipSprite();
Jump();
}
private void Run()
{
float controlThrow = CrossPlatformInputManager.GetAxis("Horizontal");//value is beteween -1 to +1
Vector2 playerVelocity = new Vector2(controlThrow * runSpeed, myRigidBody.velocity.y);
myRigidBody.velocity = playerVelocity;
print(playerVelocity);
bool playerHasHorizontalSpeed = Mathf.Abs(myRigidBody.velocity.x) > Mathf.Epsilon;
myAnimator.SetBool("Run", playerHasHorizontalSpeed);
}
private void ClimbLadder()
{
if (!myFeet.IsTouchingLayers(LayerMask.GetMask("Ladder")))
{
myAnimator.SetBool("Climb", false);
myRigidBody.gravityScale = gravityScaleAtStart;
return;
}
float controlThrow = CrossPlatformInputManager.GetAxis("Vertical");
Vector2 climbVelocity = new Vector2(myRigidBody.velocity.x, controlThrow * climbspeed );
myRigidBody.velocity = climbVelocity;
myRigidBody.gravityScale = 0f;
bool playerHasVerticalSpeed = Mathf.Abs(myRigidBody.velocity.y) > Mathf.Epsilon;
myAnimator.SetBool("Climb", playerHasVerticalSpeed);
}
private void Jump()
{
if (!myFeet.IsTouchingLayers(LayerMask.GetMask("Ground")))
return;
if (CrossPlatformInputManager.GetButtonDown("Jump"))
{
Vector2 jumpVelocityToAdd = new Vector2(0f, jumpSpeed);
myRigidBody.velocity += jumpVelocityToAdd;
}
}
private void FlipSprite()
{
bool playerHasHorizontalSpeed = Mathf.Abs(myRigidBody.velocity.x) > Mathf.Epsilon;
if (playerHasHorizontalSpeed)
{
transform.localScale = new Vector2(Mathf.Sign(myRigidBody.velocity.x), 1f);
}
}
}
‘’’
Thank you for your code. Would you mind formatting it properly to increase the readability? See this link.
And have you already compared your code to the Lecture Project Changes which can be found in the Resources of this lecture?
Hi
I tried to do exactly what it says there and it doesn’t work.
And regarding the code, I can’t find any differences.
Hello!
I tried your code and there’s nothing wrong with it. Based on your screenshots it seems like you changed something in the inspector, I can see that the movement speed of the enemy is set to 0.
As for the player, I’m not sure since you didn’t provide any screenshot, but you probably changed something there since it is working for me with no modification whatsoever. Check your settings in the inspector for both objects, and if using prefabs, check them too.
Hope that helps.
Hii!
Can you send me some screenshots with your player inspector? If you have enough time…
Thank you so much!
Unfortunately, I don’t have that particular project, so I can’t show you a player inspector.
I can see that you haven’t override your collider nor your Player script, try applying those changes to the prefab and see if that helps with your issue, if not, I’m going to ask you to do the following:
In case you haven’t, upload your project to Github in a public repo and post the link here so I can clone the project and check it, if you don’t have a GitHub account or don’t want to upload your project there, I’ll ask you to create a Unity Package by doing the following:
To create a package go to Assets > Export Package.
A window will pop up, be sure that all the folders and assets are selected. Then, at the bottom right of that window you’ll see a button that says “Export…”, click on it. That will create a package.
Upload the newly created package to a cloud drive of your choice (Google Drive, Dropbox, any other of your choice), post a public link to that package here. This way I’ll be able to test your project and see what is going on because as it is, it will be very hard to detect the issue since your code worked perfectly fine for me.
@Yee Hi!
Thank you sooo much for your help and I apologize for the delayed response. I did exactly what you taught me and here is the drive link.
My player still doesn’t jump, and the enemy doesn’t detect the objects he touches to return.
Please help me, thank you soooo much!
https://drive.google.com/drive/folders/1yCDm3pdp0USnURGuvYh2GNyO5XH1_uvs?usp=sharing
I have some bad news; the moment I opened your game it gave me like 20 errors or so, you are using deprecated code and a very old version of Cinemachine, I had to delete all of that and recompile the scripts to be able to play your game, this means that my version and your version are not the same, my solution might not work for you.
I did two things for this to work:
You can see in the gif the player can jump and that the enemy returns when finding a ledge. I think that might be your issue, the layers, if it isn’t, then I have no idea what it is, sorry.
@Yee I could update my version of unity so that your solution works … I don’t know what else to do …
Well, you can upgrade but keep it mind that doing that will throw you a bunch of errors, if you are not experienced enough looking at an all-red console is kinda intimidating.
What I recommend is to copy-paste your entire project, add it to Unity Hub and upgrade that version so you can have two games in two different versions and see if you can fix any.
@Yee
Ok. What version do you recommend?
I did not find cinemachine in another to install, and the asset package only worked on this, but I can use another
The asset package is outdated, I highly recommend not using it at all, maybe the models, but that’s it, as for the newer Cinemachine you’ll have to go to Window > Package Manager, change it to Unity Registry, you’ll find Cinemachine there.
I recommend using the latest version that is not in beta and has long term support (it is marked as LTS).
When starting a new project be sure to stick to the version the project is in, swapping between versions is dangerous, you might lose all your work, if you want to switch versions you can copy-paste the project or use version control.
@Yee
From there I also got the current cinemachine, but now I saw that there is an update.
Thank you from the bottom of my heart for all the help. This project is very important to me.
This topic was automatically closed 24 hours after the last reply. New replies are no longer allowed.