Of Lava Geysers and Thrusters
As seen in the updated video in the main post, I have finally added that lava geyser from the Level 1 block out.
It actually went through two iterations:
First, I made a box primitive, attached the same lava shader I used for the background (free from Asset Store). This actually forced me to learn a little about shaders, as since the shader asset was designed to be use globally controlled (If i changed the speed of the lava flow for one object it was attached to, it would change them all). This forced me to not only duplicate the shader, but to also go in and make minor edits to it in the code, so that it would be it’s own independent shader(basically had to change the variable names in the file, and link them in a separate script). This allowed the “ground” lava to move horizontally, while the geyser lava shader “shot upwards”. The actual rising of the geyser was done with an animation played on a loop. The timing of the geyser was baked into the animation, making it hard to change. While it looked decent enough, it was too…square. I really wanted to do something with particles. But how? I wanted that rock to shoot up and down with the lava geyser, and I wanted collisions.
The end result was a lot of work. I made two particle effects. The first was a small “bubbling” effect that let the player know the geyser was about to fire(as well as a sound cue). The second was the firing geyser particles. I kept the original box shaped geyser in, but hid it’s mesh but kept the collision shape. I then adjusted it’s animation so that the collision box would match the particles of the geyser. This was a tedious process. It involved creating key frames in the animation and matching the top (and even the bottom…you fly under the geyser as it shoots up and starts to fall down) with the particle mass.
A long with this I also decided I wanted to control the firing of the geyser outside of it’s animation. So the animation of the collision box was just one cycle,and animated and timed to match with one cycle of the particle effect.
The real challenge for me was the timing. While I knew about InvokeRepeating, that did not solve the issue that I had two particle effects that had to be played one after the other. This is where I learned about Coroutines. Through some trial and error, and some frustrated google searches I came up with this that allowed me to control the time between the lava firing (with out it spamming it which kept happening in different variations of my code)
void Start ()
{
audioSource = GetComponent<AudioSource>();
InvokeRepeating("LavaGeyserTimer", 1f, timeBetweenBursts);
}
void LavaGeyserTimer()
{
StartCoroutine(LavaActivation());
}
IEnumerator LavaActivation()
{
// Start bubbling effect and sound
lavaGeyserWarmup.Play();
audioSource.PlayOneShot(lavaGeyserBubbleAudio);
yield return new WaitForSeconds(1);
//Stop bubbling effect and start main geyser effect/sound and collision box animation
lavaGeyserWarmup.Stop();
lavaGeyserCollider.Play();
lavaGeyserMain.Play();
audioSource.PlayOneShot(lavaGeyserShootingAudio);
yield return new WaitForSeconds(3);
lavaGeyserMain.Stop();
}
What this does is on Start(), it InvokesRepeating the method that starts a Coroutine. The Invoke controls the timing between the geysers (adjustable from the editor), and the Coroutine is what does the timing to start and stop the effects in order, as well as the audio for each effect. So it starts the warmup(bubbling effect) which is 1.2 seconds long. After one second it will stop the bubbling effect and fire the geyser, which lasts three second, it then stops the effect. Since stopping the effect does not destroy the particles, the geyser naturally falls back to earth(gravity modifier on particle effect).
The actual particles are a lava texture I opened in Photoshop and feathered the edges then made a particle material in Unity.
A quick GIF of the geyser.
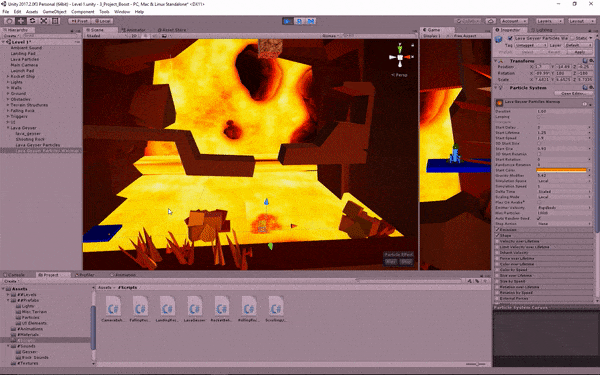
Since I am on the topic of particles, I also added my rockets thrusters. Mine is made with everything you have in Unity, no special stuff. But to get that nice point, you need to use the Size Over Lifetime to get it to dimish to a point, and have a very narrow radius cone with about 100 particles over time. I then created a light at attached it the particle gameObject, and turned off the light (unchecked it) in the component screen. Then using the Lights section I made it so the engine made a flickering light on the rocket and surrounding terrain… You can see it in the video in the main post. Here is a screen shot of my settings.
Hope you enjoy. I plan to have a prototype of the first two levels playable soon for everyone.