Here is the complete log after running this on the console:
&“D:\VideoGamesProjects\PuzzlePlatforms\Binaries\Win64\PuzzlePlatforms-Win64-Debug.exe” D:\VideoGamesProjects\PuzzlePlatforms\PuzzlePlatforms.uproject -game -log
LogInit: Build: ++UE4+Release-4.26-CL-0
LogInit: Engine Version: 4.26.2-0+++UE4+Release-4.26
LogInit: Compatible Engine Version: 4.26.0-0+++UE4+Release-4.26
LogInit: Net CL: 0
LogInit: OS: Windows 10 (Release 2009) (), CPU: Intel(R) Core(TM) i5-4690 CPU @ 3.50GHz, GPU: NVIDIA GeForce GTX 1050 Ti
LogInit: Compiled (64-bit): Nov 16 2021 20:53:11
LogInit: Compiled with Visual C++: 19.29.30137.00
LogInit: Build Configuration: Debug
LogInit: Branch Name: ++UE4+Release-4.26
LogInit: Command Line: -game -log
LogInit: Base Directory: D:/VideoGamesProjects/PuzzlePlatforms/Binaries/Win64/
LogInit: Allocator: binned2
LogInit: Installed Engine Build: 0
LogDevObjectVersion: Number of dev versions registered: 29
LogDevObjectVersion: Dev-Blueprints (B0D832E4-1F89-4F0D-ACCF-7EB736FD4AA2): 10
LogDevObjectVersion: Dev-Build (E1C64328-A22C-4D53-A36C-8E866417BD8C): 0
LogDevObjectVersion: Dev-Core (375EC13C-06E4-48FB-B500-84F0262A717E): 4
LogDevObjectVersion: Dev-Editor (E4B068ED-F494-42E9-A231-DA0B2E46BB41): 40
LogDevObjectVersion: Dev-Framework (CFFC743F-43B0-4480-9391-14DF171D2073): 37
LogDevObjectVersion: Dev-Mobile (B02B49B5-BB20-44E9-A304-32B752E40360): 3
LogDevObjectVersion: Dev-Networking (A4E4105C-59A1-49B5-A7C5-40C4547EDFEE): 0
LogDevObjectVersion: Dev-Online (39C831C9-5AE6-47DC-9A44-9C173E1C8E7C): 0
LogDevObjectVersion: Dev-Physics (78F01B33-EBEA-4F98-B9B4-84EACCB95AA2): 4
LogDevObjectVersion: Dev-Platform (6631380F-2D4D-43E0-8009-CF276956A95A): 0
LogDevObjectVersion: Dev-Rendering (12F88B9F-8875-4AFC-A67C-D90C383ABD29): 44
LogDevObjectVersion: Dev-Sequencer (7B5AE74C-D270-4C10-A958-57980B212A5A): 12
LogDevObjectVersion: Dev-VR (D7296918-1DD6-4BDD-9DE2-64A83CC13884): 3
LogDevObjectVersion: Dev-LoadTimes (C2A15278-BFE7-4AFE-6C17-90FF531DF755): 1
LogDevObjectVersion: Private-Geometry (6EACA3D4-40EC-4CC1-B786-8BED09428FC5): 3
LogDevObjectVersion: Dev-AnimPhys (29E575DD-E0A3-4627-9D10-D276232CDCEA): 17
LogDevObjectVersion: Dev-Anim (AF43A65D-7FD3-4947-9873-3E8ED9C1BB05): 15
LogDevObjectVersion: Dev-ReflectionCapture (6B266CEC-1EC7-4B8F-A30B-E4D90942FC07): 1
LogDevObjectVersion: Dev-Automation (0DF73D61-A23F-47EA-B727-89E90C41499A): 1
LogDevObjectVersion: FortniteMain (601D1886-AC64-4F84-AA16-D3DE0DEAC7D6): 43
LogDevObjectVersion: FortniteRelease (E7086368-6B23-4C58-8439-1B7016265E91): 1
LogDevObjectVersion: Dev-Enterprise (9DFFBCD6-494F-0158-E221-12823C92A888): 10
LogDevObjectVersion: Dev-Niagara (F2AED0AC-9AFE-416F-8664-AA7FFA26D6FC): 1
LogDevObjectVersion: Dev-Destruction (174F1F0B-B4C6-45A5-B13F-2EE8D0FB917D): 10
LogDevObjectVersion: Dev-Physics-Ext (35F94A83-E258-406C-A318-09F59610247C): 40
LogDevObjectVersion: Dev-PhysicsMaterial-Chaos (B68FC16E-8B1B-42E2-B453-215C058844FE): 1
LogDevObjectVersion: Dev-CineCamera (B2E18506-4273-CFC2-A54E-F4BB758BBA07): 1
LogDevObjectVersion: Dev-VirtualProduction (64F58936-FD1B-42BA-BA96-7289D5D0FA4E): 1
LogDevObjectVersion: Dev-MediaFramework (6F0ED827-A609-4895-9C91-998D90180EA4): 2
LogInit: Presizing for max 2097152 objects, including 1 objects not considered by GC, pre-allocating 0 bytes for permanent pool.
LogConfig: Applying CVar settings from Section [/Script/Engine.StreamingSettings] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Engine.ini]
LogConfig: Setting CVar [[s.MinBulkDataSizeForAsyncLoading:131072]]
LogConfig: Setting CVar [[s.AsyncLoadingThreadEnabled:0]]
LogConfig: Setting CVar [[s.EventDrivenLoaderEnabled:1]]
LogConfig: Setting CVar [[s.WarnIfTimeLimitExceeded:0]]
LogConfig: Setting CVar [[s.TimeLimitExceededMultiplier:1.5]]
LogConfig: Setting CVar [[s.TimeLimitExceededMinTime:0.005]]
LogConfig: Setting CVar [[s.UseBackgroundLevelStreaming:1]]
LogConfig: Setting CVar [[s.PriorityAsyncLoadingExtraTime:15.0]]
LogConfig: Setting CVar [[s.LevelStreamingActorsUpdateTimeLimit:5.0]]
LogConfig: Setting CVar [[s.PriorityLevelStreamingActorsUpdateExtraTime:5.0]]
LogConfig: Setting CVar [[s.LevelStreamingComponentsRegistrationGranularity:10]]
LogConfig: Setting CVar [[s.UnregisterComponentsTimeLimit:1.0]]
LogConfig: Setting CVar [[s.LevelStreamingComponentsUnregistrationGranularity:5]]
LogConfig: Setting CVar [[s.FlushStreamingOnExit:1]]
LogStreaming: Display: Async Loading initialized: Event Driven Loader: true, Async Loading Thread: false, Async Post Load: true
LogInit: Object subsystem initialized
LogConfig: Setting CVar [[con.DebugEarlyDefault:1]]
LogConfig: Setting CVar [[r.setres:1280x720]]
LogConfig: Setting CVar [[fx.NiagaraAllowRuntimeScalabilityChanges:1]]
[2021.11.20-19.25.47:530][ 0]LogConfig: Setting CVar [[con.DebugEarlyDefault:1]]
[2021.11.20-19.25.47:530][ 0]LogConfig: Setting CVar [[r.setres:1280x720]]
[2021.11.20-19.25.47:532][ 0]LogConfig: Setting CVar [[fx.NiagaraAllowRuntimeScalabilityChanges:1]]
[2021.11.20-19.25.47:620][ 0]LogConfig: Applying CVar settings from Section [/Script/Engine.RendererSettings] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Engine.ini]
[2021.11.20-19.25.47:621][ 0]LogConfig: Setting CVar [[r.GPUCrashDebugging:0]]
[2021.11.20-19.25.47:625][ 0]LogConfig: Applying CVar settings from Section [/Script/Engine.RendererOverrideSettings] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Engine.ini]
[2021.11.20-19.25.47:626][ 0]LogConfig: Applying CVar settings from Section [/Script/Engine.StreamingSettings] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Engine.ini]
[2021.11.20-19.25.47:627][ 0]LogConfig: Setting CVar [[s.MinBulkDataSizeForAsyncLoading:131072]]
[2021.11.20-19.25.47:628][ 0]LogConfig: Setting CVar [[s.AsyncLoadingThreadEnabled:0]]
[2021.11.20-19.25.47:629][ 0]LogConfig: Setting CVar [[s.EventDrivenLoaderEnabled:1]]
[2021.11.20-19.25.47:630][ 0]LogConfig: Setting CVar [[s.WarnIfTimeLimitExceeded:0]]
[2021.11.20-19.25.47:632][ 0]LogConfig: Setting CVar [[s.TimeLimitExceededMultiplier:1.5]]
[2021.11.20-19.25.47:633][ 0]LogConfig: Setting CVar [[s.TimeLimitExceededMinTime:0.005]]
[2021.11.20-19.25.47:634][ 0]LogConfig: Setting CVar [[s.UseBackgroundLevelStreaming:1]]
[2021.11.20-19.25.47:635][ 0]LogConfig: Setting CVar [[s.PriorityAsyncLoadingExtraTime:15.0]]
[2021.11.20-19.25.47:636][ 0]LogConfig: Setting CVar [[s.LevelStreamingActorsUpdateTimeLimit:5.0]]
[2021.11.20-19.25.47:637][ 0]LogConfig: Setting CVar [[s.PriorityLevelStreamingActorsUpdateExtraTime:5.0]]
[2021.11.20-19.25.47:642][ 0]LogConfig: Setting CVar [[s.LevelStreamingComponentsRegistrationGranularity:10]]
[2021.11.20-19.25.47:643][ 0]LogConfig: Setting CVar [[s.UnregisterComponentsTimeLimit:1.0]]
[2021.11.20-19.25.47:644][ 0]LogConfig: Setting CVar [[s.LevelStreamingComponentsUnregistrationGranularity:5]]
[2021.11.20-19.25.47:645][ 0]LogConfig: Setting CVar [[s.FlushStreamingOnExit:1]]
[2021.11.20-19.25.47:646][ 0]LogConfig: Applying CVar settings from Section [/Script/Engine.GarbageCollectionSettings] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Engine.ini]
[2021.11.20-19.25.47:648][ 0]LogConfig: Setting CVar [[gc.MaxObjectsNotConsideredByGC:1]]
[2021.11.20-19.25.47:649][ 0]LogConfig: Setting CVar [[gc.SizeOfPermanentObjectPool:0]]
[2021.11.20-19.25.47:650][ 0]LogConfig: Setting CVar [[gc.FlushStreamingOnGC:0]]
[2021.11.20-19.25.47:651][ 0]LogConfig: Setting CVar [[gc.NumRetriesBeforeForcingGC:10]]
[2021.11.20-19.25.47:652][ 0]LogConfig: Setting CVar [[gc.AllowParallelGC:1]]
[2021.11.20-19.25.47:654][ 0]LogConfig: Setting CVar [[gc.TimeBetweenPurgingPendingKillObjects:61.1]]
[2021.11.20-19.25.47:655][ 0]LogConfig: Setting CVar [[gc.MaxObjectsInEditor:25165824]]
[2021.11.20-19.25.47:655][ 0]LogConfig: Setting CVar [[gc.IncrementalBeginDestroyEnabled:1]]
[2021.11.20-19.25.47:657][ 0]LogConfig: Setting CVar [[gc.CreateGCClusters:1]]
[2021.11.20-19.25.47:658][ 0]LogConfig: Setting CVar [[gc.MinGCClusterSize:5]]
[2021.11.20-19.25.47:658][ 0]LogConfig: Setting CVar [[gc.ActorClusteringEnabled:0]]
[2021.11.20-19.25.47:659][ 0]LogConfig: Setting CVar [[gc.BlueprintClusteringEnabled:0]]
[2021.11.20-19.25.47:660][ 0]LogConfig: Setting CVar [[gc.UseDisregardForGCOnDedicatedServers:0]]
[2021.11.20-19.25.47:661][ 0]LogConfig: Setting CVar [[gc.MultithreadedDestructionEnabled:1]]
[2021.11.20-19.25.47:661][ 0]LogConfig: Applying CVar settings from Section [/Script/Engine.NetworkSettings] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Engine.ini]
[2021.11.20-19.25.48:036][ 0]LogConfig: Applying CVar settings from Section [ViewDistanceQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:036][ 0]LogConfig: Setting CVar [[r.SkeletalMeshLODBias:0]]
[2021.11.20-19.25.48:039][ 0]LogConfig: Setting CVar [[r.ViewDistanceScale:1.0]]
[2021.11.20-19.25.48:040][ 0]LogConfig: Applying CVar settings from Section [AntiAliasingQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:042][ 0]LogConfig: Setting CVar [[r.PostProcessAAQuality:4]]
[2021.11.20-19.25.48:043][ 0]LogConfig: Applying CVar settings from Section [ShadowQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:044][ 0]LogConfig: Setting CVar [[r.LightFunctionQuality:1]]
[2021.11.20-19.25.48:046][ 0]LogConfig: Setting CVar [[r.ShadowQuality:5]]
[2021.11.20-19.25.48:047][ 0]LogConfig: Setting CVar [[r.Shadow.CSM.MaxCascades:10]]
[2021.11.20-19.25.48:048][ 0]LogConfig: Setting CVar [[r.Shadow.MaxResolution:2048]]
[2021.11.20-19.25.48:049][ 0]LogConfig: Setting CVar [[r.Shadow.MaxCSMResolution:2048]]
[2021.11.20-19.25.48:051][ 0]LogConfig: Setting CVar [[r.Shadow.RadiusThreshold:0.01]]
[2021.11.20-19.25.48:052][ 0]LogConfig: Setting CVar [[r.Shadow.DistanceScale:1.0]]
[2021.11.20-19.25.48:054][ 0]LogConfig: Setting CVar [[r.Shadow.CSM.TransitionScale:1.0]]
[2021.11.20-19.25.48:060][ 0]LogConfig: Setting CVar [[r.Shadow.PreShadowResolutionFactor:1.0]]
[2021.11.20-19.25.48:062][ 0]LogConfig: Setting CVar [[r.DistanceFieldShadowing:1]]
[2021.11.20-19.25.48:064][ 0]LogConfig: Setting CVar [[r.DistanceFieldAO:1]]
[2021.11.20-19.25.48:065][ 0]LogConfig: Setting CVar [[r.AOQuality:2]]
[2021.11.20-19.25.48:066][ 0]LogConfig: Setting CVar [[r.VolumetricFog:1]]
[2021.11.20-19.25.48:067][ 0]LogConfig: Setting CVar [[r.VolumetricFog.GridPixelSize:8]]
[2021.11.20-19.25.48:069][ 0]LogConfig: Setting CVar [[r.VolumetricFog.GridSizeZ:128]]
[2021.11.20-19.25.48:070][ 0]LogConfig: Setting CVar [[r.VolumetricFog.HistoryMissSupersampleCount:4]]
[2021.11.20-19.25.48:072][ 0]LogConfig: Setting CVar [[r.LightMaxDrawDistanceScale:1]]
[2021.11.20-19.25.48:074][ 0]LogConfig: Setting CVar [[r.CapsuleShadows:1]]
[2021.11.20-19.25.48:075][ 0]LogConfig: Applying CVar settings from Section [PostProcessQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:076][ 0]LogConfig: Setting CVar [[r.MotionBlurQuality:4]]
[2021.11.20-19.25.48:077][ 0]LogConfig: Setting CVar [[r.AmbientOcclusionMipLevelFactor:0.4]]
[2021.11.20-19.25.48:079][ 0]LogConfig: Setting CVar [[r.AmbientOcclusionMaxQuality:100]]
[2021.11.20-19.25.48:080][ 0]LogConfig: Setting CVar [[r.AmbientOcclusionLevels:-1]]
[2021.11.20-19.25.48:081][ 0]LogConfig: Setting CVar [[r.AmbientOcclusionRadiusScale:1.0]]
[2021.11.20-19.25.48:082][ 0]LogConfig: Setting CVar [[r.DepthOfFieldQuality:2]]
[2021.11.20-19.25.48:083][ 0]LogConfig: Setting CVar [[r.RenderTargetPoolMin:400]]
[2021.11.20-19.25.48:084][ 0]LogConfig: Setting CVar [[r.LensFlareQuality:2]]
[2021.11.20-19.25.48:087][ 0]LogConfig: Setting CVar [[r.SceneColorFringeQuality:1]]
[2021.11.20-19.25.48:087][ 0]LogConfig: Setting CVar [[r.EyeAdaptationQuality:2]]
[2021.11.20-19.25.48:089][ 0]LogConfig: Setting CVar [[r.BloomQuality:5]]
[2021.11.20-19.25.48:090][ 0]LogConfig: Setting CVar [[r.FastBlurThreshold:100]]
[2021.11.20-19.25.48:091][ 0]LogConfig: Setting CVar [[r.Upscale.Quality:3]]
[2021.11.20-19.25.48:092][ 0]LogConfig: Setting CVar [[r.Tonemapper.GrainQuantization:1]]
[2021.11.20-19.25.48:094][ 0]LogConfig: Setting CVar [[r.LightShaftQuality:1]]
[2021.11.20-19.25.48:095][ 0]LogConfig: Setting CVar [[r.Filter.SizeScale:1]]
[2021.11.20-19.25.48:096][ 0]LogConfig: Setting CVar [[r.Tonemapper.Quality:5]]
[2021.11.20-19.25.48:097][ 0]LogConfig: Setting CVar [[r.DOF.Gather.AccumulatorQuality:1 ; higher gathering accumulator quality]]
[2021.11.20-19.25.48:098][ 0]LogConfig: Setting CVar [[r.DOF.Gather.PostfilterMethod:1 ; Median3x3 postfilering method]]
[2021.11.20-19.25.48:099][ 0]LogConfig: Setting CVar [[r.DOF.Gather.EnableBokehSettings:0 ; no bokeh simulation when gathering]]
[2021.11.20-19.25.48:103][ 0]LogConfig: Setting CVar [[r.DOF.Gather.RingCount:4 ; medium number of samples when gathering]]
[2021.11.20-19.25.48:104][ 0]LogConfig: Setting CVar [[r.DOF.Scatter.ForegroundCompositing:1 ; additive foreground scattering]]
[2021.11.20-19.25.48:105][ 0]LogConfig: Setting CVar [[r.DOF.Scatter.BackgroundCompositing:2 ; additive background scattering]]
[2021.11.20-19.25.48:106][ 0]LogConfig: Setting CVar [[r.DOF.Scatter.EnableBokehSettings:1 ; bokeh simulation when scattering]]
[2021.11.20-19.25.48:108][ 0]LogConfig: Setting CVar [[r.DOF.Scatter.MaxSpriteRatio:0.1 ; only a maximum of 10% of scattered bokeh]]
[2021.11.20-19.25.48:109][ 0]LogConfig: Setting CVar [[r.DOF.Recombine.Quality:1 ; cheap slight out of focus]]
[2021.11.20-19.25.48:110][ 0]LogConfig: Setting CVar [[r.DOF.Recombine.EnableBokehSettings:0 ; no bokeh simulation on slight out of focus]]
[2021.11.20-19.25.48:111][ 0]LogConfig: Setting CVar [[r.DOF.TemporalAAQuality:1 ; more stable temporal accumulation]]
[2021.11.20-19.25.48:112][ 0]LogConfig: Setting CVar [[r.DOF.Kernel.MaxForegroundRadius:0.025]]
[2021.11.20-19.25.48:113][ 0]LogConfig: Setting CVar [[r.DOF.Kernel.MaxBackgroundRadius:0.025]]
[2021.11.20-19.25.48:114][ 0]LogConfig: Applying CVar settings from Section [TextureQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:115][ 0]LogConfig: Setting CVar [[r.Streaming.MipBias:0]]
[2021.11.20-19.25.48:118][ 0]LogConfig: Setting CVar [[r.Streaming.AmortizeCPUToGPUCopy:0]]
[2021.11.20-19.25.48:119][ 0]LogConfig: Setting CVar [[r.Streaming.MaxNumTexturesToStreamPerFrame:0]]
[2021.11.20-19.25.48:121][ 0]LogConfig: Setting CVar [[r.Streaming.Boost:1]]
[2021.11.20-19.25.48:122][ 0]LogConfig: Setting CVar [[r.MaxAnisotropy:8]]
[2021.11.20-19.25.48:123][ 0]LogConfig: Setting CVar [[r.VT.MaxAnisotropy:8]]
[2021.11.20-19.25.48:124][ 0]LogConfig: Setting CVar [[r.Streaming.LimitPoolSizeToVRAM:0]]
[2021.11.20-19.25.48:125][ 0]LogConfig: Setting CVar [[r.Streaming.PoolSize:1000]]
[2021.11.20-19.25.48:126][ 0]LogConfig: Setting CVar [[r.Streaming.MaxEffectiveScreenSize:0]]
[2021.11.20-19.25.48:127][ 0]LogConfig: Applying CVar settings from Section [EffectsQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:128][ 0]LogConfig: Setting CVar [[r.TranslucencyLightingVolumeDim:64]]
[2021.11.20-19.25.48:129][ 0]LogConfig: Setting CVar [[r.RefractionQuality:2]]
[2021.11.20-19.25.48:130][ 0]LogConfig: Setting CVar [[r.SSR.Quality:3]]
[2021.11.20-19.25.48:131][ 0]LogConfig: Setting CVar [[r.SSR.HalfResSceneColor:0]]
[2021.11.20-19.25.48:133][ 0]LogConfig: Setting CVar [[r.SceneColorFormat:4]]
[2021.11.20-19.25.48:136][ 0]LogConfig: Setting CVar [[r.DetailMode:2]]
[2021.11.20-19.25.48:137][ 0]LogConfig: Setting CVar [[r.TranslucencyVolumeBlur:1]]
[2021.11.20-19.25.48:138][ 0]LogConfig: Setting CVar [[r.MaterialQualityLevel:1 ; High quality]]
[2021.11.20-19.25.48:139][ 0]LogConfig: Setting CVar [[r.AnisotropicMaterials:1]]
[2021.11.20-19.25.48:141][ 0]LogConfig: Setting CVar [[r.SSS.Scale:1]]
[2021.11.20-19.25.48:142][ 0]LogConfig: Setting CVar [[r.SSS.SampleSet:2]]
[2021.11.20-19.25.48:143][ 0]LogConfig: Setting CVar [[r.SSS.Quality:1]]
[2021.11.20-19.25.48:144][ 0]LogConfig: Setting CVar [[r.SSS.HalfRes:0]]
[2021.11.20-19.25.48:145][ 0]LogConfig: Setting CVar [[r.SSGI.Quality:3]]
[2021.11.20-19.25.48:146][ 0]LogConfig: Setting CVar [[r.EmitterSpawnRateScale:1.0]]
[2021.11.20-19.25.48:147][ 0]LogConfig: Setting CVar [[r.ParticleLightQuality:2]]
[2021.11.20-19.25.48:150][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.AerialPerspectiveLUT.FastApplyOnOpaque:1 ; Always have FastSkyLUT 1 in this case to avoid wrong sky]]
[2021.11.20-19.25.48:151][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.AerialPerspectiveLUT.SampleCountMaxPerSlice:4]]
[2021.11.20-19.25.48:152][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.AerialPerspectiveLUT.DepthResolution:16.0]]
[2021.11.20-19.25.48:152][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.FastSkyLUT:1]]
[2021.11.20-19.25.48:153][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.FastSkyLUT.SampleCountMin:4.0]]
[2021.11.20-19.25.48:153][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.FastSkyLUT.SampleCountMax:128.0]]
[2021.11.20-19.25.48:154][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.SampleCountMin:4.0]]
[2021.11.20-19.25.48:155][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.SampleCountMax:128.0]]
[2021.11.20-19.25.48:155][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.TransmittanceLUT.UseSmallFormat:0]]
[2021.11.20-19.25.48:155][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.TransmittanceLUT.SampleCount:10.0]]
[2021.11.20-19.25.48:156][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.MultiScatteringLUT.SampleCount:15.0]]
[2021.11.20-19.25.48:156][ 0]LogConfig: Setting CVar [[fx.Niagara.QualityLevel:3]]
[2021.11.20-19.25.48:160][ 0]LogConfig: Applying CVar settings from Section [FoliageQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:160][ 0]LogConfig: Setting CVar [[foliage.DensityScale:1.0]]
[2021.11.20-19.25.48:161][ 0]LogConfig: Setting CVar [[grass.DensityScale:1.0]]
[2021.11.20-19.25.48:163][ 0]LogConfig: Applying CVar settings from Section [ShadingQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:166][ 0]LogConfig: Setting CVar [[r.HairStrands.SkyLighting.IntegrationType:2]]
[2021.11.20-19.25.48:167][ 0]LogConfig: Setting CVar [[r.HairStrands.SkyAO.SampleCount:4]]
[2021.11.20-19.25.48:168][ 0]LogConfig: Setting CVar [[r.HairStrands.Visibility.MSAA.SamplePerPixel:4]]
[2021.11.20-19.25.48:281][ 0]LogInit: Selected Device Profile: [WindowsNoEditor]
[2021.11.20-19.25.48:301][ 0]LogInit: Applying CVar settings loaded from the selected device profile: [WindowsNoEditor]
[2021.11.20-19.25.48:363][ 0]LogHAL: Display: Platform has ~ 16 GB [17054134272 / 17179869184 / 16], which maps to Larger [LargestMinGB=32, LargerMinGB=12, DefaultMinGB=8, SmallerMinGB=6, SmallestMinGB=0)
[2021.11.20-19.25.48:377][ 0]LogInit: Going up to parent DeviceProfile [Windows]
[2021.11.20-19.25.48:377][ 0]LogInit: Going up to parent DeviceProfile []
[2021.11.20-19.25.48:440][ 0]LogConfig: Applying CVar settings from Section [ViewDistanceQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:440][ 0]LogConfig: Setting CVar [[r.SkeletalMeshLODBias:0]]
[2021.11.20-19.25.48:444][ 0]LogConfig: Setting CVar [[r.ViewDistanceScale:1.0]]
[2021.11.20-19.25.48:445][ 0]LogConfig: Applying CVar settings from Section [AntiAliasingQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:447][ 0]LogConfig: Setting CVar [[r.PostProcessAAQuality:4]]
[2021.11.20-19.25.48:448][ 0]LogConfig: Applying CVar settings from Section [ShadowQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:449][ 0]LogConfig: Setting CVar [[r.LightFunctionQuality:1]]
[2021.11.20-19.25.48:451][ 0]LogConfig: Setting CVar [[r.ShadowQuality:5]]
[2021.11.20-19.25.48:452][ 0]LogConfig: Setting CVar [[r.Shadow.CSM.MaxCascades:10]]
[2021.11.20-19.25.48:454][ 0]LogConfig: Setting CVar [[r.Shadow.MaxResolution:2048]]
[2021.11.20-19.25.48:455][ 0]LogConfig: Setting CVar [[r.Shadow.MaxCSMResolution:2048]]
[2021.11.20-19.25.48:457][ 0]LogConfig: Setting CVar [[r.Shadow.RadiusThreshold:0.01]]
[2021.11.20-19.25.48:460][ 0]LogConfig: Setting CVar [[r.Shadow.DistanceScale:1.0]]
[2021.11.20-19.25.48:461][ 0]LogConfig: Setting CVar [[r.Shadow.CSM.TransitionScale:1.0]]
[2021.11.20-19.25.48:461][ 0]LogConfig: Setting CVar [[r.Shadow.PreShadowResolutionFactor:1.0]]
[2021.11.20-19.25.48:462][ 0]LogConfig: Setting CVar [[r.DistanceFieldShadowing:1]]
[2021.11.20-19.25.48:464][ 0]LogConfig: Setting CVar [[r.DistanceFieldAO:1]]
[2021.11.20-19.25.48:465][ 0]LogConfig: Setting CVar [[r.AOQuality:2]]
[2021.11.20-19.25.48:467][ 0]LogConfig: Setting CVar [[r.VolumetricFog:1]]
[2021.11.20-19.25.48:469][ 0]LogConfig: Setting CVar [[r.VolumetricFog.GridPixelSize:8]]
[2021.11.20-19.25.48:470][ 0]LogConfig: Setting CVar [[r.VolumetricFog.GridSizeZ:128]]
[2021.11.20-19.25.48:472][ 0]LogConfig: Setting CVar [[r.VolumetricFog.HistoryMissSupersampleCount:4]]
[2021.11.20-19.25.48:474][ 0]LogConfig: Setting CVar [[r.LightMaxDrawDistanceScale:1]]
[2021.11.20-19.25.48:477][ 0]LogConfig: Setting CVar [[r.CapsuleShadows:1]]
[2021.11.20-19.25.48:477][ 0]LogConfig: Applying CVar settings from Section [PostProcessQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:478][ 0]LogConfig: Setting CVar [[r.MotionBlurQuality:4]]
[2021.11.20-19.25.48:480][ 0]LogConfig: Setting CVar [[r.AmbientOcclusionMipLevelFactor:0.4]]
[2021.11.20-19.25.48:481][ 0]LogConfig: Setting CVar [[r.AmbientOcclusionMaxQuality:100]]
[2021.11.20-19.25.48:482][ 0]LogConfig: Setting CVar [[r.AmbientOcclusionLevels:-1]]
[2021.11.20-19.25.48:483][ 0]LogConfig: Setting CVar [[r.AmbientOcclusionRadiusScale:1.0]]
[2021.11.20-19.25.48:485][ 0]LogConfig: Setting CVar [[r.DepthOfFieldQuality:2]]
[2021.11.20-19.25.48:486][ 0]LogConfig: Setting CVar [[r.RenderTargetPoolMin:400]]
[2021.11.20-19.25.48:487][ 0]LogConfig: Setting CVar [[r.LensFlareQuality:2]]
[2021.11.20-19.25.48:489][ 0]LogConfig: Setting CVar [[r.SceneColorFringeQuality:1]]
[2021.11.20-19.25.48:492][ 0]LogConfig: Setting CVar [[r.EyeAdaptationQuality:2]]
[2021.11.20-19.25.48:492][ 0]LogConfig: Setting CVar [[r.BloomQuality:5]]
[2021.11.20-19.25.48:494][ 0]LogConfig: Setting CVar [[r.FastBlurThreshold:100]]
[2021.11.20-19.25.48:495][ 0]LogConfig: Setting CVar [[r.Upscale.Quality:3]]
[2021.11.20-19.25.48:495][ 0]LogConfig: Setting CVar [[r.Tonemapper.GrainQuantization:1]]
[2021.11.20-19.25.48:496][ 0]LogConfig: Setting CVar [[r.LightShaftQuality:1]]
[2021.11.20-19.25.48:498][ 0]LogConfig: Setting CVar [[r.Filter.SizeScale:1]]
[2021.11.20-19.25.48:499][ 0]LogConfig: Setting CVar [[r.Tonemapper.Quality:5]]
[2021.11.20-19.25.48:499][ 0]LogConfig: Setting CVar [[r.DOF.Gather.AccumulatorQuality:1 ; higher gathering accumulator quality]]
[2021.11.20-19.25.48:501][ 0]LogConfig: Setting CVar [[r.DOF.Gather.PostfilterMethod:1 ; Median3x3 postfilering method]]
[2021.11.20-19.25.48:502][ 0]LogConfig: Setting CVar [[r.DOF.Gather.EnableBokehSettings:0 ; no bokeh simulation when gathering]]
[2021.11.20-19.25.48:503][ 0]LogConfig: Setting CVar [[r.DOF.Gather.RingCount:4 ; medium number of samples when gathering]]
[2021.11.20-19.25.48:506][ 0]LogConfig: Setting CVar [[r.DOF.Scatter.ForegroundCompositing:1 ; additive foreground scattering]]
[2021.11.20-19.25.48:506][ 0]LogConfig: Setting CVar [[r.DOF.Scatter.BackgroundCompositing:2 ; additive background scattering]]
[2021.11.20-19.25.48:507][ 0]LogConfig: Setting CVar [[r.DOF.Scatter.EnableBokehSettings:1 ; bokeh simulation when scattering]]
[2021.11.20-19.25.48:508][ 0]LogConfig: Setting CVar [[r.DOF.Scatter.MaxSpriteRatio:0.1 ; only a maximum of 10% of scattered bokeh]]
[2021.11.20-19.25.48:509][ 0]LogConfig: Setting CVar [[r.DOF.Recombine.Quality:1 ; cheap slight out of focus]]
[2021.11.20-19.25.48:511][ 0]LogConfig: Setting CVar [[r.DOF.Recombine.EnableBokehSettings:0 ; no bokeh simulation on slight out of focus]]
[2021.11.20-19.25.48:512][ 0]LogConfig: Setting CVar [[r.DOF.TemporalAAQuality:1 ; more stable temporal accumulation]]
[2021.11.20-19.25.48:513][ 0]LogConfig: Setting CVar [[r.DOF.Kernel.MaxForegroundRadius:0.025]]
[2021.11.20-19.25.48:514][ 0]LogConfig: Setting CVar [[r.DOF.Kernel.MaxBackgroundRadius:0.025]]
[2021.11.20-19.25.48:515][ 0]LogConfig: Applying CVar settings from Section [TextureQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:516][ 0]LogConfig: Setting CVar [[r.Streaming.MipBias:0]]
[2021.11.20-19.25.48:517][ 0]LogConfig: Setting CVar [[r.Streaming.AmortizeCPUToGPUCopy:0]]
[2021.11.20-19.25.48:518][ 0]LogConfig: Setting CVar [[r.Streaming.MaxNumTexturesToStreamPerFrame:0]]
[2021.11.20-19.25.48:520][ 0]LogConfig: Setting CVar [[r.Streaming.Boost:1]]
[2021.11.20-19.25.48:523][ 0]LogConfig: Setting CVar [[r.MaxAnisotropy:8]]
[2021.11.20-19.25.48:525][ 0]LogConfig: Setting CVar [[r.VT.MaxAnisotropy:8]]
[2021.11.20-19.25.48:526][ 0]LogConfig: Setting CVar [[r.Streaming.LimitPoolSizeToVRAM:0]]
[2021.11.20-19.25.48:527][ 0]LogConfig: Setting CVar [[r.Streaming.PoolSize:1000]]
[2021.11.20-19.25.48:528][ 0]LogConfig: Setting CVar [[r.Streaming.MaxEffectiveScreenSize:0]]
[2021.11.20-19.25.48:529][ 0]LogConfig: Applying CVar settings from Section [EffectsQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:530][ 0]LogConfig: Setting CVar [[r.TranslucencyLightingVolumeDim:64]]
[2021.11.20-19.25.48:532][ 0]LogConfig: Setting CVar [[r.RefractionQuality:2]]
[2021.11.20-19.25.48:533][ 0]LogConfig: Setting CVar [[r.SSR.Quality:3]]
[2021.11.20-19.25.48:534][ 0]LogConfig: Setting CVar [[r.SSR.HalfResSceneColor:0]]
[2021.11.20-19.25.48:536][ 0]LogConfig: Setting CVar [[r.SceneColorFormat:4]]
[2021.11.20-19.25.48:536][ 0]LogConfig: Setting CVar [[r.DetailMode:2]]
[2021.11.20-19.25.48:537][ 0]LogConfig: Setting CVar [[r.TranslucencyVolumeBlur:1]]
[2021.11.20-19.25.48:537][ 0]LogConfig: Setting CVar [[r.MaterialQualityLevel:1 ; High quality]]
[2021.11.20-19.25.48:538][ 0]LogConfig: Setting CVar [[r.AnisotropicMaterials:1]]
[2021.11.20-19.25.48:539][ 0]LogConfig: Setting CVar [[r.SSS.Scale:1]]
[2021.11.20-19.25.48:541][ 0]LogConfig: Setting CVar [[r.SSS.SampleSet:2]]
[2021.11.20-19.25.48:542][ 0]LogConfig: Setting CVar [[r.SSS.Quality:1]]
[2021.11.20-19.25.48:543][ 0]LogConfig: Setting CVar [[r.SSS.HalfRes:0]]
[2021.11.20-19.25.48:544][ 0]LogConfig: Setting CVar [[r.SSGI.Quality:3]]
[2021.11.20-19.25.48:544][ 0]LogConfig: Setting CVar [[r.EmitterSpawnRateScale:1.0]]
[2021.11.20-19.25.48:545][ 0]LogConfig: Setting CVar [[r.ParticleLightQuality:2]]
[2021.11.20-19.25.48:546][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.AerialPerspectiveLUT.FastApplyOnOpaque:1 ; Always have FastSkyLUT 1 in this case to avoid wrong sky]]
[2021.11.20-19.25.48:547][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.AerialPerspectiveLUT.SampleCountMaxPerSlice:4]]
[2021.11.20-19.25.48:547][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.AerialPerspectiveLUT.DepthResolution:16.0]]
[2021.11.20-19.25.48:548][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.FastSkyLUT:1]]
[2021.11.20-19.25.48:550][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.FastSkyLUT.SampleCountMin:4.0]]
[2021.11.20-19.25.48:550][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.FastSkyLUT.SampleCountMax:128.0]]
[2021.11.20-19.25.48:553][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.SampleCountMin:4.0]]
[2021.11.20-19.25.48:554][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.SampleCountMax:128.0]]
[2021.11.20-19.25.48:554][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.TransmittanceLUT.UseSmallFormat:0]]
[2021.11.20-19.25.48:555][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.TransmittanceLUT.SampleCount:10.0]]
[2021.11.20-19.25.48:556][ 0]LogConfig: Setting CVar [[r.SkyAtmosphere.MultiScatteringLUT.SampleCount:15.0]]
[2021.11.20-19.25.48:557][ 0]LogConfig: Setting CVar [[fx.Niagara.QualityLevel:3]]
[2021.11.20-19.25.48:558][ 0]LogConfig: Applying CVar settings from Section [FoliageQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:559][ 0]LogConfig: Setting CVar [[foliage.DensityScale:1.0]]
[2021.11.20-19.25.48:560][ 0]LogConfig: Setting CVar [[grass.DensityScale:1.0]]
[2021.11.20-19.25.48:561][ 0]LogConfig: Applying CVar settings from Section [ShadingQuality@3] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Scalability.ini]
[2021.11.20-19.25.48:561][ 0]LogConfig: Setting CVar [[r.HairStrands.SkyLighting.IntegrationType:2]]
[2021.11.20-19.25.48:562][ 0]LogConfig: Setting CVar [[r.HairStrands.SkyAO.SampleCount:4]]
[2021.11.20-19.25.48:563][ 0]LogConfig: Setting CVar [[r.HairStrands.Visibility.MSAA.SamplePerPixel:4]]
[2021.11.20-19.25.48:564][ 0]LogConfig: Applying CVar settings from Section [Startup] File [../../../../Repositorios/UnrealEngine/Engine/Config/ConsoleVariables.ini]
[2021.11.20-19.25.48:591][ 0]LogConfig: Setting CVar [[net.UseAdaptiveNetUpdateFrequency:0]]
[2021.11.20-19.25.48:591][ 0]LogConfig: Setting CVar [[p.chaos.AllowCreatePhysxBodies:1]]
[2021.11.20-19.25.48:592][ 0]LogConfig: Setting CVar [[fx.SkipVectorVMBackendOptimizations:1]]
[2021.11.20-19.25.48:593][ 0]LogConfig: Applying CVar settings from Section [ConsoleVariables] File [D:/VideoGamesProjects/PuzzlePlatforms/Saved/Config/WindowsNoEditor/Engine.ini]
[2021.11.20-19.25.49:228][ 0]LogInit: Computer: DESKTOP-LOR7EIH
[2021.11.20-19.25.49:228][ 0]LogInit: User: king
[2021.11.20-19.25.49:231][ 0]LogInit: CPU Page size=4096, Cores=4
[2021.11.20-19.25.49:234][ 0]LogInit: High frequency timer resolution =10.000000 MHz
[2021.11.20-19.25.49:236][ 0]LogMemory: Memory total: Physical=15.9GB (16GB approx)
[2021.11.20-19.25.49:238][ 0]LogMemory: Platform Memory Stats for WindowsNoEditor
[2021.11.20-19.25.49:240][ 0]LogMemory: Process Physical Memory: 98.52 MB used, 98.55 MB peak
[2021.11.20-19.25.49:243][ 0]LogMemory: Process Virtual Memory: 77.10 MB used, 77.10 MB peak
[2021.11.20-19.25.49:244][ 0]LogMemory: Physical Memory: 8153.06 MB used, 8111.03 MB free, 16264.09 MB total
[2021.11.20-19.25.49:245][ 0]LogMemory: Virtual Memory: 134212680.00 MB used, 5049.92 MB free, 134217728.00 MB total
[2021.11.20-19.25.49:253][ 0]LogWindows: WindowsPlatformFeatures enabled
[2021.11.20-19.25.55:849][ 0]LogInit: Physics initialised using underlying interface: PhysX
[2021.11.20-19.25.55:966][ 0]LogInit: Using OS detected language (es-ES).
[2021.11.20-19.25.55:966][ 0]LogInit: Using OS detected locale (es-ES).
[2021.11.20-19.25.56:223][ 0]LogTextLocalizationManager: No specific localization for 'es-ES' exists, so the 'es' localization will be used.
[2021.11.20-19.25.57:222][ 0]LogWindowsTextInputMethodSystem: Display: IME system deactivated.
[2021.11.20-19.25.58:862][ 0]LogSlate: New Slate User Created. User Index 0, Is Virtual User: 0
[2021.11.20-19.25.58:865][ 0]LogSlate: Slate User Registered. User Index 0, Is Virtual User: 0
[2021.11.20-19.25.59:473][ 0]LogHMD: Failed to initialize OpenVR with code 110
[2021.11.20-19.25.59:473][ 0]LogD3D11RHI: D3D11 min allowed feature level: 11_0
[2021.11.20-19.25.59:476][ 0]LogD3D11RHI: D3D11 max allowed feature level: 11_0
[2021.11.20-19.25.59:478][ 0]LogD3D11RHI: D3D11 adapters:
[2021.11.20-19.25.59:892][ 0]LogD3D11RHI: 0. 'NVIDIA GeForce GTX 1050 Ti' (Feature Level 11_0)
[2021.11.20-19.25.59:925][ 0]LogD3D11RHI: 4018/0/8132 MB DedicatedVideo/DedicatedSystem/SharedSystem, Outputs:1, VendorId:0x10de
[2021.11.20-19.25.59:959][ 0]LogD3D11RHI: 1. 'Intel(R) HD Graphics 4600' (Feature Level 11_0)
[2021.11.20-19.25.59:960][ 0]LogD3D11RHI: 112/0/2048 MB DedicatedVideo/DedicatedSystem/SharedSystem, Outputs:0, VendorId:0x8086
[2021.11.20-19.25.59:982][ 0]LogD3D11RHI: 2. 'Microsoft Basic Render Driver' (Feature Level 11_0)
[2021.11.20-19.25.59:983][ 0]LogD3D11RHI: 0/0/8132 MB DedicatedVideo/DedicatedSystem/SharedSystem, Outputs:0, VendorId:0x1414
[2021.11.20-19.25.59:984][ 0]LogD3D11RHI: Chosen D3D11 Adapter: 0
[2021.11.20-19.26.00:169][ 0]LogD3D11RHI: Creating new Direct3DDevice
[2021.11.20-19.26.00:170][ 0]LogD3D11RHI: GPU DeviceId: 0x1c82 (for the marketing name, search the web for "GPU Device Id")
[2021.11.20-19.26.00:171][ 0]LogWindows: EnumDisplayDevices:
[2021.11.20-19.26.00:171][ 0]LogWindows: 0. 'Intel(R) HD Graphics 4600' (P:0 D:0)
[2021.11.20-19.26.00:172][ 0]LogWindows: 1. 'Intel(R) HD Graphics 4600' (P:0 D:0)
[2021.11.20-19.26.00:173][ 0]LogWindows: 2. 'Intel(R) HD Graphics 4600' (P:0 D:0)
[2021.11.20-19.26.00:173][ 0]LogWindows: 3. 'NVIDIA GeForce GTX 1050 Ti' (P:1 D:1)
[2021.11.20-19.26.00:174][ 0]LogWindows: 4. 'NVIDIA GeForce GTX 1050 Ti' (P:0 D:0)
[2021.11.20-19.26.00:175][ 0]LogWindows: 5. 'NVIDIA GeForce GTX 1050 Ti' (P:0 D:0)
[2021.11.20-19.26.00:176][ 0]LogWindows: 6. 'NVIDIA GeForce GTX 1050 Ti' (P:0 D:0)
[2021.11.20-19.26.00:176][ 0]LogWindows: DebugString: PrimaryIsNotTheChoosenAdapter PrimaryIsNotTheChoosenAdapter PrimaryIsNotTheChoosenAdapter FoundDriverCount:4
[2021.11.20-19.26.00:176][ 0]LogD3D11RHI: Adapter Name: NVIDIA GeForce GTX 1050 Ti
[2021.11.20-19.26.00:177][ 0]LogD3D11RHI: Driver Version: 471.96 (internal:30.0.14.7196, unified:471.96)
[2021.11.20-19.26.00:177][ 0]LogD3D11RHI: Driver Date: 8-27-2021
[2021.11.20-19.26.00:182][ 0]LogRHI: Texture pool is 2812 MB (70% of 4018 MB)
[2021.11.20-19.26.00:355][ 0]LogD3D11RHI: RHI has support for 64 bit atomics
[2021.11.20-19.26.00:441][ 0]LogD3D11RHI: Async texture creation enabled
[2021.11.20-19.26.00:442][ 0]LogD3D11RHI: Array index from any shader is supported
[2021.11.20-19.26.01:913][ 0]LogD3D11RHI: GPU Timing Frequency: 1000.000000 (Debug: 2 1)
[2021.11.20-19.26.01:969][ 0]LogRendererCore: Ray tracing is disabled. Reason: r.RayTracing=0.
[2021.11.20-19.26.02:267][ 0]LogShaderLibrary: Display: Using ../../Content/ShaderArchive-Global-PCD3D_SM5.ushaderbytecode for material shader code. Total 2081 unique shaders.
[2021.11.20-19.26.02:368][ 0]LogShaderLibrary: Display: Cooked Context: Using Shared Shader Library Global
[2021.11.20-19.26.02:404][ 0]LogTemp: Warning: Clearing the OS Cache
[2021.11.20-19.26.05:355][ 0]LogSlate: Using FreeType 2.10.0
[2021.11.20-19.26.05:754][ 0]LogSlate: SlateFontServices - WITH_FREETYPE: 1, WITH_HARFBUZZ: 1
[2021.11.20-19.26.09:704][ 0]LogShaderLibrary: Display: Using ../../Content/ShaderArchive-PuzzlePlatforms-PCD3D_SM5.ushaderbytecode for material shader code. Total 1482 unique shaders.
[2021.11.20-19.26.09:709][ 0]LogShaderLibrary: Display: Cooked Context: Using Shared Shader Library PuzzlePlatforms
[2021.11.20-19.26.09:740][ 0]LogRHI: Display: Opened pipeline cache after state change and enqueued 0 of 0 tasks for precompile.
[2021.11.20-19.26.09:766][ 0]LogRHI: Display: Failed to open default shader pipeline cache for PuzzlePlatforms using shader platform 0.
[2021.11.20-19.26.09:767][ 0]LogInit: Using OS detected language (es-ES).
[2021.11.20-19.26.09:768][ 0]LogInit: Using OS detected locale (es-ES).
[2021.11.20-19.26.09:768][ 0]LogTextLocalizationManager: No localization for 'es-ES' exists, so 'en' will be used for the language.
[2021.11.20-19.26.09:768][ 0]LogTextLocalizationManager: No localization for 'es-ES' exists, so 'en' will be used for the locale.
[2021.11.20-19.26.09:775][ 0]LogSlate: FontCache flush requested. Reason: Culture for localization was changed
[2021.11.20-19.26.09:775][ 0]LogSlate: FontCache flush requested. Reason: Culture for localization was changed
[2021.11.20-19.26.09:897][ 0]LogTextLocalizationManager: Compacting localization data took 0.02ms
[2021.11.20-19.26.10:497][ 0]LogAssetRegistry: FAssetRegistry took 0.4821 seconds to start up
[2021.11.20-19.26.15:468][ 0]LogStreaming: Display: FlushAsyncLoading: 1 QueuedPackages, 0 AsyncPackages
[2021.11.20-19.26.15:697][ 0]LogPackageLocalizationCache: Processed 21 localized package path(s) for 1 prioritized culture(s) in 0.006960 seconds
[2021.11.20-19.26.16:147][ 0]LogSerialization: Display: AllowBulkDataInIoStore: 'true'
[2021.11.20-19.26.16:231][ 0]LogInit: Selected Device Profile: [WindowsNoEditor]
[2021.11.20-19.26.16:261][ 0]LogInit: Active device profile: [0000024099B48C80][0000024099B7EC50 49] WindowsNoEditor
[2021.11.20-19.26.16:261][ 0]LogInit: Profiles: [0000024099B48800][000002408C780010 49] Windows, [0000024099B48C80][0000024099B7EC50 49] WindowsNoEditor,
[2021.11.20-19.26.18:236][ 0]LogNetVersion: PuzzlePlatforms 1.0.0, NetCL: 0, EngineNetVer: 16, GameNetVer: 0 (Checksum: 1210254132)
[2021.11.20-19.26.27:402][ 0]LogAudioCaptureCore: Display: No Audio Capture implementations found. Audio input will be silent.
[2021.11.20-19.26.27:403][ 0]LogAudioCaptureCore: Display: No Audio Capture implementations found. Audio input will be silent.
[2021.11.20-19.26.27:444][ 0]LogHMD: PokeAHoleMaterial loaded successfully
[2021.11.20-19.26.27:854][ 0]LogStreaming: Error: Couldn't find file for package /Game/MenuSystem/WBP_ConnectionCandidate requested by async loading code. NameToLoad: /Game/MenuSystem/WBP_ConnectionCandidate
[2021.11.20-19.26.27:901][ 0]LogStreaming: Error: Found 0 dependent packages...
[2021.11.20-19.26.27:947][ 0]Error: CDO Constructor (ConnectionMenu): Failed to find /Game/MenuSystem/WBP_ConnectionCandidate.WBP_ConnectionCandidate_C
[2021.11.20-19.26.27:952][ 0]Error: CDO Constructor (ConnectionMenu): Failed to find /Game/MenuSystem/WBP_ConnectionCandidate.WBP_ConnectionCandidate_C
[2021.11.20-19.26.53:103][ 0]LogStats: FPlatformStackWalk::StackWalkAndDump - 25.117 s
[2021.11.20-19.26.53:104][ 0]LogOutputDevice: Error: === Handled ensure: ===
[2021.11.20-19.26.53:104][ 0]LogOutputDevice: Error:
[2021.11.20-19.26.53:107][ 0]LogOutputDevice: Error: Ensure condition failed: ConnectionCandidateFinder.Class != nullptr [File:D:\VideoGamesProjects\PuzzlePlatforms\Source\PuzzlePlatforms\MenuSystem\ConnectionMenu.cpp] [Line: 14]
[2021.11.20-19.26.53:107][ 0]LogOutputDevice: Error:
[2021.11.20-19.26.53:108][ 0]LogOutputDevice: Error: Stack:
[2021.11.20-19.26.53:108][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6d0117c01 PuzzlePlatforms-Win64-Debug.exe!FDebug::OptionallyLogFormattedEnsureMessageReturningFalseImpl() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Core\Private\Misc\AssertionMacros.cpp:497]
[2021.11.20-19.26.53:109][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6c77f9ca4 PuzzlePlatforms-Win64-Debug.exe!<lambda_73afea6066107c5a3c5772d0062d2186>::operator()() [D:\VideoGamesProjects\PuzzlePlatforms\Source\PuzzlePlatforms\MenuSystem\ConnectionMenu.cpp:14]
[2021.11.20-19.26.53:109][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6c77f9638 PuzzlePlatforms-Win64-Debug.exe!DispatchCheckVerify<bool,<lambda_73afea6066107c5a3c5772d0062d2186> >() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Core\Public\Misc\AssertionMacros.h:165]
[2021.11.20-19.26.53:110][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cfb396a4 PuzzlePlatforms-Win64-Debug.exe!UConnectionMenu::UConnectionMenu() [D:\VideoGamesProjects\PuzzlePlatforms\Source\PuzzlePlatforms\MenuSystem\ConnectionMenu.cpp:14]
[2021.11.20-19.26.53:110][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cfb50eac PuzzlePlatforms-Win64-Debug.exe!UConnectionMenu::__DefaultConstructor() [D:\VideoGamesProjects\PuzzlePlatforms\Source\PuzzlePlatforms\MenuSystem\ConnectionMenu.h:26]
[2021.11.20-19.26.53:111][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cfb5045c PuzzlePlatforms-Win64-Debug.exe!InternalConstructor<UConnectionMenu>() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\CoreUObject\Public\UObject\Class.h:3308]
[2021.11.20-19.26.53:111][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6d0ab7c38 PuzzlePlatforms-Win64-Debug.exe!UClass::CreateDefaultObject() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\CoreUObject\Private\UObject\Class.cpp:3707]
[2021.11.20-19.26.53:112][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6d10d716b PuzzlePlatforms-Win64-Debug.exe!UObjectLoadAllCompiledInDefaultProperties() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\CoreUObject\Private\UObject\UObjectBase.cpp:908]
[2021.11.20-19.26.53:112][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6d1083fc4 PuzzlePlatforms-Win64-Debug.exe!ProcessNewlyLoadedUObjects() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\CoreUObject\Private\UObject\UObjectBase.cpp:997]
[2021.11.20-19.26.53:113][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd038ebd PuzzlePlatforms-Win64-Debug.exe!FEngineLoop::PreInitPostStartupScreen() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\LaunchEngineLoop.cpp:3017]
[2021.11.20-19.26.53:113][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd0350af PuzzlePlatforms-Win64-Debug.exe!FEngineLoop::PreInit() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\LaunchEngineLoop.cpp:3591]
[2021.11.20-19.26.53:114][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd01aa6b PuzzlePlatforms-Win64-Debug.exe!EnginePreInit() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\Launch.cpp:42]
[2021.11.20-19.26.53:114][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd02838f PuzzlePlatforms-Win64-Debug.exe!GuardedMain() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\Launch.cpp:127]
[2021.11.20-19.26.53:115][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd0714e3 PuzzlePlatforms-Win64-Debug.exe!WinMain() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\Windows\LaunchWindows.cpp:257]
[2021.11.20-19.26.53:115][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6dd3f0a12 PuzzlePlatforms-Win64-Debug.exe!__scrt_common_main_seh() [d:\a01\_work\6\s\src\vctools\crt\vcstartup\src\startup\exe_common.inl:288]
[2021.11.20-19.26.53:116][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff9be277034 KERNEL32.DLL!UnknownFunction []
[2021.11.20-19.26.53:116][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff9bee82651 ntdll.dll!UnknownFunction []
[2021.11.20-19.26.53:116][ 0]LogOutputDevice: Error:
[2021.11.20-19.26.53:154][ 0]LogStats: SubmitErrorReport - 0.000 s
[2021.11.20-19.26.53:553][ 0]LogStats: SendNewReport - 0.399 s
[2021.11.20-19.26.53:553][ 0]LogStats: FDebug::EnsureFailed - 25.588 s
[2021.11.20-19.26.54:493][ 0]LogStreaming: Error: Couldn't find file for package /Game/MenuSystem/WBP_ConnectionMenu requested by async loading code. NameToLoad: /Game/MenuSystem/WBP_ConnectionMenu
[2021.11.20-19.26.54:494][ 0]LogStreaming: Error: Found 0 dependent packages...
[2021.11.20-19.26.54:495][ 0]Error: CDO Constructor (PuzzlePlatformsGameInstance): Failed to find /Game/MenuSystem/WBP_ConnectionMenu.WBP_ConnectionMenu_C
[2021.11.20-19.26.54:496][ 0]Error: CDO Constructor (PuzzlePlatformsGameInstance): Failed to find /Game/MenuSystem/WBP_ConnectionMenu.WBP_ConnectionMenu_C
[2021.11.20-19.26.54:891][ 0]LogStats: FPlatformStackWalk::StackWalkAndDump - 0.390 s
[2021.11.20-19.26.54:892][ 0]LogOutputDevice: Error: === Handled ensure: ===
[2021.11.20-19.26.54:893][ 0]LogOutputDevice: Error:
[2021.11.20-19.26.54:894][ 0]LogOutputDevice: Error: Ensure condition failed: ConnectionMenu.Class != nullptr [File:D:\VideoGamesProjects\PuzzlePlatforms\Source\PuzzlePlatforms\PuzzlePlatformsGameInstance.cpp] [Line: 21]
[2021.11.20-19.26.54:895][ 0]LogOutputDevice: Error:
[2021.11.20-19.26.54:895][ 0]LogOutputDevice: Error: Stack:
[2021.11.20-19.26.54:896][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6d0117c01 PuzzlePlatforms-Win64-Debug.exe!FDebug::OptionallyLogFormattedEnsureMessageReturningFalseImpl() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Core\Private\Misc\AssertionMacros.cpp:497]
[2021.11.20-19.26.54:896][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6c77fba3c PuzzlePlatforms-Win64-Debug.exe!<lambda_fd91518b8cbd1468bf206a5f24225f5c>::operator()() [D:\VideoGamesProjects\PuzzlePlatforms\Source\PuzzlePlatforms\PuzzlePlatformsGameInstance.cpp:21]
[2021.11.20-19.26.54:897][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6c77fb358 PuzzlePlatforms-Win64-Debug.exe!DispatchCheckVerify<bool,<lambda_fd91518b8cbd1468bf206a5f24225f5c> >() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Core\Public\Misc\AssertionMacros.h:165]
[2021.11.20-19.26.54:897][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cfb497ab PuzzlePlatforms-Win64-Debug.exe!UPuzzlePlatformsGameInstance::UPuzzlePlatformsGameInstance() [D:\VideoGamesProjects\PuzzlePlatforms\Source\PuzzlePlatforms\PuzzlePlatformsGameInstance.cpp:21]
[2021.11.20-19.26.54:898][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cfb5326c PuzzlePlatforms-Win64-Debug.exe!UPuzzlePlatformsGameInstance::__DefaultConstructor() [D:\VideoGamesProjects\PuzzlePlatforms\Source\PuzzlePlatforms\PuzzlePlatformsGameInstance.h:17]
[2021.11.20-19.26.54:898][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cfb52f1c PuzzlePlatforms-Win64-Debug.exe!InternalConstructor<UPuzzlePlatformsGameInstance>() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\CoreUObject\Public\UObject\Class.h:3308]
[2021.11.20-19.26.54:899][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6d0ab7c38 PuzzlePlatforms-Win64-Debug.exe!UClass::CreateDefaultObject() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\CoreUObject\Private\UObject\Class.cpp:3707]
[2021.11.20-19.26.54:899][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6d10d716b PuzzlePlatforms-Win64-Debug.exe!UObjectLoadAllCompiledInDefaultProperties() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\CoreUObject\Private\UObject\UObjectBase.cpp:908]
[2021.11.20-19.26.54:900][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6d1083fc4 PuzzlePlatforms-Win64-Debug.exe!ProcessNewlyLoadedUObjects() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\CoreUObject\Private\UObject\UObjectBase.cpp:997]
[2021.11.20-19.26.54:900][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd038ebd PuzzlePlatforms-Win64-Debug.exe!FEngineLoop::PreInitPostStartupScreen() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\LaunchEngineLoop.cpp:3017]
[2021.11.20-19.26.54:901][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd0350af PuzzlePlatforms-Win64-Debug.exe!FEngineLoop::PreInit() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\LaunchEngineLoop.cpp:3591]
[2021.11.20-19.26.54:901][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd01aa6b PuzzlePlatforms-Win64-Debug.exe!EnginePreInit() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\Launch.cpp:42]
[2021.11.20-19.26.54:902][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd02838f PuzzlePlatforms-Win64-Debug.exe!GuardedMain() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\Launch.cpp:127]
[2021.11.20-19.26.54:902][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd0714e3 PuzzlePlatforms-Win64-Debug.exe!WinMain() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\Windows\LaunchWindows.cpp:257]
[2021.11.20-19.26.54:906][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6dd3f0a12 PuzzlePlatforms-Win64-Debug.exe!__scrt_common_main_seh() [d:\a01\_work\6\s\src\vctools\crt\vcstartup\src\startup\exe_common.inl:288]
[2021.11.20-19.26.54:906][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff9be277034 KERNEL32.DLL!UnknownFunction []
[2021.11.20-19.26.54:907][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff9bee82651 ntdll.dll!UnknownFunction []
[2021.11.20-19.26.54:907][ 0]LogOutputDevice: Error:
[2021.11.20-19.26.54:942][ 0]LogStats: SubmitErrorReport - 0.000 s
[2021.11.20-19.26.55:022][ 0]LogStats: SendNewReport - 0.080 s
[2021.11.20-19.26.55:022][ 0]LogStats: FDebug::EnsureFailed - 0.522 s
[2021.11.20-19.26.56:356][ 0]LogUObjectBase: Warning: -------------- Default Property warnings and errors:
[2021.11.20-19.26.56:356][ 0]LogUObjectBase: Warning: Error: CDO Constructor (ConnectionMenu): Failed to find /Game/MenuSystem/WBP_ConnectionCandidate.WBP_ConnectionCandidate_C
[2021.11.20-19.26.56:357][ 0]LogUObjectBase: Warning: Error: CDO Constructor (PuzzlePlatformsGameInstance): Failed to find /Game/MenuSystem/WBP_ConnectionMenu.WBP_ConnectionMenu_C
After the last messages the same window alert pops up.
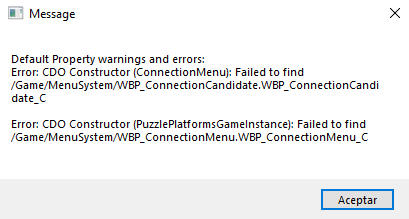
After acepting that message, the console adds the following:
[2021.11.20-19.26.54:891][ 0]LogStats: FPlatformStackWalk::StackWalkAndDump - 0.390 s
[2021.11.20-19.26.54:892][ 0]LogOutputDevice: Error: === Handled ensure: ===
[2021.11.20-19.26.54:893][ 0]LogOutputDevice: Error:
[2021.11.20-19.26.54:894][ 0]LogOutputDevice: Error: Ensure condition failed: ConnectionMenu.Class != nullptr [File:D:\VideoGamesProjects\PuzzlePlatforms\Source\PuzzlePlatforms\PuzzlePlatformsGameInstance.cpp] [Line: 21]
[2021.11.20-19.26.54:895][ 0]LogOutputDevice: Error:
[2021.11.20-19.26.54:895][ 0]LogOutputDevice: Error: Stack:
[2021.11.20-19.26.54:896][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6d0117c01 PuzzlePlatforms-Win64-Debug.exe!FDebug::OptionallyLogFormattedEnsureMessageReturningFalseImpl() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Core\Private\Misc\AssertionMacros.cpp:497]
[2021.11.20-19.26.54:896][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6c77fba3c PuzzlePlatforms-Win64-Debug.exe!<lambda_fd91518b8cbd1468bf206a5f24225f5c>::operator()() [D:\VideoGamesProjects\PuzzlePlatforms\Source\PuzzlePlatforms\PuzzlePlatformsGameInstance.cpp:21]
[2021.11.20-19.26.54:897][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6c77fb358 PuzzlePlatforms-Win64-Debug.exe!DispatchCheckVerify<bool,<lambda_fd91518b8cbd1468bf206a5f24225f5c> >() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Core\Public\Misc\AssertionMacros.h:165]
[2021.11.20-19.26.54:897][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cfb497ab PuzzlePlatforms-Win64-Debug.exe!UPuzzlePlatformsGameInstance::UPuzzlePlatformsGameInstance() [D:\VideoGamesProjects\PuzzlePlatforms\Source\PuzzlePlatforms\PuzzlePlatformsGameInstance.cpp:21]
[2021.11.20-19.26.54:898][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cfb5326c PuzzlePlatforms-Win64-Debug.exe!UPuzzlePlatformsGameInstance::__DefaultConstructor() [D:\VideoGamesProjects\PuzzlePlatforms\Source\PuzzlePlatforms\PuzzlePlatformsGameInstance.h:17]
[2021.11.20-19.26.54:898][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cfb52f1c PuzzlePlatforms-Win64-Debug.exe!InternalConstructor<UPuzzlePlatformsGameInstance>() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\CoreUObject\Public\UObject\Class.h:3308]
[2021.11.20-19.26.54:899][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6d0ab7c38 PuzzlePlatforms-Win64-Debug.exe!UClass::CreateDefaultObject() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\CoreUObject\Private\UObject\Class.cpp:3707]
[2021.11.20-19.26.54:899][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6d10d716b PuzzlePlatforms-Win64-Debug.exe!UObjectLoadAllCompiledInDefaultProperties() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\CoreUObject\Private\UObject\UObjectBase.cpp:908]
[2021.11.20-19.26.54:900][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6d1083fc4 PuzzlePlatforms-Win64-Debug.exe!ProcessNewlyLoadedUObjects() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\CoreUObject\Private\UObject\UObjectBase.cpp:997]
[2021.11.20-19.26.54:900][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd038ebd PuzzlePlatforms-Win64-Debug.exe!FEngineLoop::PreInitPostStartupScreen() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\LaunchEngineLoop.cpp:3017]
[2021.11.20-19.26.54:901][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd0350af PuzzlePlatforms-Win64-Debug.exe!FEngineLoop::PreInit() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\LaunchEngineLoop.cpp:3591]
[2021.11.20-19.26.54:901][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd01aa6b PuzzlePlatforms-Win64-Debug.exe!EnginePreInit() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\Launch.cpp:42]
[2021.11.20-19.26.54:902][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd02838f PuzzlePlatforms-Win64-Debug.exe!GuardedMain() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\Launch.cpp:127]
[2021.11.20-19.26.54:902][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6cd0714e3 PuzzlePlatforms-Win64-Debug.exe!WinMain() [D:\Repositorios\UnrealEngine\Engine\Source\Runtime\Launch\Private\Windows\LaunchWindows.cpp:257]
[2021.11.20-19.26.54:906][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff6dd3f0a12 PuzzlePlatforms-Win64-Debug.exe!__scrt_common_main_seh() [d:\a01\_work\6\s\src\vctools\crt\vcstartup\src\startup\exe_common.inl:288]
[2021.11.20-19.26.54:906][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff9be277034 KERNEL32.DLL!UnknownFunction []
[2021.11.20-19.26.54:907][ 0]LogOutputDevice: Error: [Callstack] 0x00007ff9bee82651 ntdll.dll!UnknownFunction []
[2021.11.20-19.26.54:907][ 0]LogOutputDevice: Error:
[2021.11.20-19.26.54:942][ 0]LogStats: SubmitErrorReport - 0.000 s
[2021.11.20-19.26.55:022][ 0]LogStats: SendNewReport - 0.080 s
[2021.11.20-19.26.55:022][ 0]LogStats: FDebug::EnsureFailed - 0.522 s
[2021.11.20-19.26.56:356][ 0]LogUObjectBase: Warning: -------------- Default Property warnings and errors:
[2021.11.20-19.26.56:356][ 0]LogUObjectBase: Warning: Error: CDO Constructor (ConnectionMenu): Failed to find /Game/MenuSystem/WBP_ConnectionCandidate.WBP_ConnectionCandidate_C
[2021.11.20-19.26.56:357][ 0]LogUObjectBase: Warning: Error: CDO Constructor (PuzzlePlatformsGameInstance): Failed to find /Game/MenuSystem/WBP_ConnectionMenu.WBP_ConnectionMenu_C
[2021.11.20-19.29.10:452][ 0]Message dialog closed, result: Ok, title: Message, text: Default Property warnings and errors:
Error: CDO Constructor (ConnectionMenu): Failed to find /Game/MenuSystem/WBP_ConnectionCandidate.WBP_ConnectionCandidate_C
Error: CDO Constructor (PuzzlePlatformsGameInstance): Failed to find /Game/MenuSystem/WBP_ConnectionMenu.WBP_ConnectionMenu_C
[2021.11.20-19.29.10:862][ 0]LogMoviePlayer: Initializing movie player
[2021.11.20-19.29.11:333][ 0]LogAndroidPermission: UAndroidPermissionCallbackProxy::GetInstance
[2021.11.20-19.29.11:379][ 0]LogOcInput: OculusInput pre-init called
[2021.11.20-19.29.11:424][ 0]LogUObjectArray: 15428 objects as part of root set at end of initial load.
[2021.11.20-19.29.11:425][ 0]LogUObjectArray: 8 objects are not in the root set, but can never be destroyed because they are in the DisregardForGC set.
[2021.11.20-19.29.11:435][ 0]LogUObjectAllocator: 3205272 out of 0 bytes used by permanent object pool.
[2021.11.20-19.29.11:438][ 0]LogUObjectArray: CloseDisregardForGC: 15428/15428 objects in disregard for GC pool
[2021.11.20-19.29.11:615][ 0]LogEngine: Initializing Engine...
[2021.11.20-19.29.11:671][ 0]LogHMD: Failed to initialize OpenVR with code 110
[2021.11.20-19.29.11:767][ 0]LogStats: UGameplayTagsManager::InitializeManager - 0.016 s
[2021.11.20-19.29.12:736][ 0]LogInit: Initializing FReadOnlyCVARCache
[2021.11.20-19.29.12:743][ 0]LogAudio: Display: Initializing Audio Device Manager...
[2021.11.20-19.29.12:767][ 0]LogAudio: Display: Audio Device Manager Initialized
[2021.11.20-19.29.12:769][ 0]LogAudio: Display: Creating Audio Device: Id: 1, Scope: Shared, Realtime: True
[2021.11.20-19.29.12:832][ 0]LogAudioMixer: Display: Audio Mixer Platform Settings:
[2021.11.20-19.29.12:832][ 0]LogAudioMixer: Display: Sample Rate: 48000
[2021.11.20-19.29.12:836][ 0]LogAudioMixer: Display: Callback Buffer Frame Size Requested: 1024
[2021.11.20-19.29.12:838][ 0]LogAudioMixer: Display: Callback Buffer Frame Size To Use: 1024
[2021.11.20-19.29.12:839][ 0]LogAudioMixer: Display: Number of buffers to queue: 2
[2021.11.20-19.29.12:839][ 0]LogAudioMixer: Display: Max Channels (voices): 32
[2021.11.20-19.29.12:841][ 0]LogAudioMixer: Display: Number of Async Source Workers: 0
[2021.11.20-19.29.12:844][ 0]LogAudio: Display: AudioDevice MaxSources: 32
[2021.11.20-19.29.12:884][ 0]LogAudio: Display: Using built-in audio occlusion.
[2021.11.20-19.29.12:885][ 0]LogAudioMixer: Display: Initializing audio mixer.
[2021.11.20-19.29.13:137][ 0]LogAudioDebug: Display: Lib vorbis DLL was dynamically loaded.
[2021.11.20-19.29.13:202][ 0]LogAudioMixer: Display: 0: FrontLeft
[2021.11.20-19.29.13:203][ 0]LogAudioMixer: Display: 1: FrontRight
[2021.11.20-19.29.13:206][ 0]LogAudioMixer: Display: 2: FrontCenter
[2021.11.20-19.29.13:207][ 0]LogAudioMixer: Display: 3: LowFrequency
[2021.11.20-19.29.13:209][ 0]LogAudioMixer: Display: 4: BackLeft
[2021.11.20-19.29.13:212][ 0]LogAudioMixer: Display: 5: BackRight
[2021.11.20-19.29.13:306][ 0]LogAudioMixer: Display: Using Audio Device Altavoces (Realtek High Definition Audio)
[2021.11.20-19.29.13:328][ 0]LogAudioMixer: Display: Initializing Sound Submixes...
[2021.11.20-19.29.13:413][ 0]LogAudioMixer: Display: Creating Master Submix 'MasterSubmixDefault'
[2021.11.20-19.29.13:414][ 0]LogAudioMixer: Display: Creating Master Submix 'MasterReverbSubmixDefault'
[2021.11.20-19.29.13:428][ 0]LogAudioMixer: Display: Creating Master Submix 'MasterEQSubmixDefault'
[2021.11.20-19.29.13:429][ 0]LogAudioMixer: FMixerPlatformXAudio2::StartAudioStream() called
[2021.11.20-19.29.13:429][ 0]LogAudioMixer: Display: Output buffers initialized: Frames=1024, Channels=6, Samples=6144
[2021.11.20-19.29.13:449][ 0]LogAudioMixer: Display: Starting AudioMixerPlatformInterface::RunInternal()
[2021.11.20-19.29.13:449][ 0]LogAudioMixer: Display: FMixerPlatformXAudio2::SubmitBuffer() called for the first time
[2021.11.20-19.29.13:449][ 0]LogInit: FAudioDevice initialized.
[2021.11.20-19.29.13:579][ 0]LogAudioMixer: Display: Audio Buffer Underrun (starvation) detected.
[2021.11.20-19.29.13:579][ 0]LogNetVersion: Set ProjectVersion to 1.0.0.0. Version Checksum will be recalculated on next use.
[2021.11.20-19.29.13:608][ 0]LogInit: Texture streaming: Enabled
[2021.11.20-19.29.13:846][ 0]Error: CDO Constructor (PuzzlePlatformsGameInstance): Failed to find /Game/MenuSystem/WBP_ConnectionMenu.WBP_ConnectionMenu_C
[2021.11.20-19.29.13:852][ 0]Error: CDO Constructor (PuzzlePlatformsGameInstance): Failed to find /Game/MenuSystem/WBP_ConnectionMenu.WBP_ConnectionMenu_C
After that an additional alert pops up and crashes instantly closing itself and the console.