Hi, thanks again for the advice. I’m still pretty stumped by this!
Here’s a new picture of Goober and his colliders:
It also shows the platforms tilemap with its green line showing the collision boundary that I think is right. The player can’t pass through that edge, only Goober. And if I change Goober to a dynamic not kinematic rigidbody he collides with the wall. (But he doesn’t turn round I guess because the trigger isn’t activated)
This is the collision matrix:
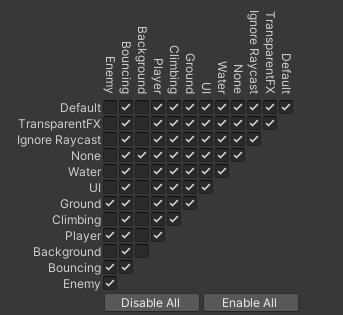
My naming has been a bit idiosyncratic unfortunately so while the tilemap is called platforms, the layer its on is called ground.
I’m not sure how you disable a layer, but I went through all the tilemaps and un-ticked their renderer components to make them disappear. The elements disappeared as expected - I think the tiles are correct.
I have been adding Debug.Log() to my code as I’ve been trying different things, and not once has it been called, so there’s definitely something there preventing it. When I switched the RigidBody to Dynamic, I also tried using onCollisionEnter2D, but that didn’t seem to get called either. At the risk of making this comment really long, I’ve pasted the code below. I’ve checked it against the Github link in the lesson, and aside from me using different variable names, it looks the same to me.
Again, thank you!
public class EnemyMovement : MonoBehaviour
{
Rigidbody2D EnemyRigidBody;
[SerializeField] float EnemySpeed = 1f;
void Start()
{
EnemyRigidBody = GetComponent<Rigidbody2D>();
}
void Update()
{
EnemyRigidBody.velocity = new Vector2 (EnemySpeed, 0f);
}
//void onCollisionEnter2D(Collision2D Collision)
//{
// Debug.Log("Collided");
//}
void onTriggerEnter2D(BoxCollider2D Collider)
{
Debug.Log("Entered trigger");
}
void onTriggerExit2D(BoxCollider2D Collider)
{
Debug.Log("Exited trigger");
EnemySpeed = -EnemySpeed;
FlipEnemyDirection();
}
void FlipEnemyDirection()
{
transform.localScale = new Vector2 (-(Mathf.Sign(EnemyRigidBody.velocity.x)), 1f);
}
}