So I am having a weird issue right now where I see this:
In the photo, you can also see what my enemy has on it.
And here is the wave:
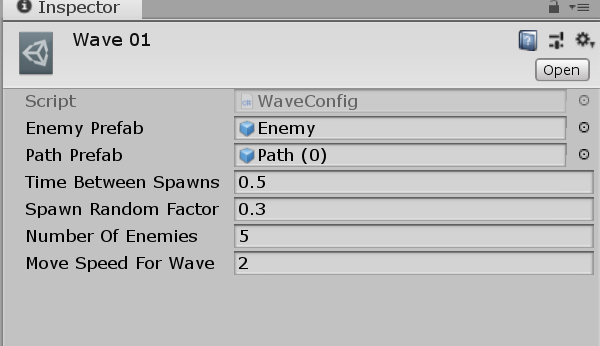
Here is the wave spawner script:
[SerializeField] GameObject enemyPrefab;
[SerializeField] GameObject pathPrefab;
[SerializeField] float timeBetweenSpawns = 0.5f;
[SerializeField] float spawnRandomFactor = 0.3f;
[SerializeField] int numberOfEnemies = 5;
[SerializeField] float moveSpeedForWave = 2f;
// The get methods for each of the serialize fields above
public GameObject GetEnemyPrefab() { return enemyPrefab; }
public List<Transform> GetWaypoints()
{
var waveWaypoints = new List<Transform>();
foreach (Transform child in pathPrefab.transform)
{
waveWaypoints.Add(child);
}
return waveWaypoints;
}
public float GetTimeBetweenSpawns() { return timeBetweenSpawns; }
public float GetSpawnRandomFactor() { return spawnRandomFactor; }
public int GetNumberOfEnemies() { return numberOfEnemies; }
public float GetMoveSpeedForWave() { return moveSpeedForWave; }
// end the get methods
The enemy pathing:
[SerializeField] WaveConfig waveConfig;
// for the wayponts
List<Transform> waypoints;
// for moving the enemies
[SerializeField] float movingSpeed = 2f;
int waypointIndex = 0;
// Start is called before the first frame update
void Start()
{
waypoints = waveConfig.GetWaypoints();
transform.position = waypoints[waypointIndex].transform.position;
}
// Update is called once per frame
void Update()
{
Move();
}
private void Move()
{
// to move the position
if (waypointIndex <= waypoints.Count - 1)
{
var targetPosition = waypoints[waypointIndex].transform.position;
var movementThisFrame = movingSpeed * Time.deltaTime;
transform.position = Vector2.MoveTowards
(transform.position, targetPosition, movementThisFrame);
// if at the last waypoint
if (transform.position == targetPosition)
{
waypointIndex++;
}
}
// no vaild waypoint
else
{
Destroy(gameObject);
}
}
And finally the enemy spawner
[SerializeField] List<WaveConfig> waveConfigs;
// to start the waves
int startingWave = 0;
// Use this for initialization
void Start()
{
var currentWave = waveConfigs[startingWave];
StartCoroutine(SpawnAllEnemiesInWave(currentWave));
}
private IEnumerator SpawnAllEnemiesInWave(WaveConfig waveConfig)
{
for (int enemyCount = 0;
enemyCount < waveConfig.GetNumberOfEnemies();
enemyCount++)
{
Instantiate(
waveConfig.GetEnemyPrefab(),
waveConfig.GetWaypoints()[0].transform.position,
Quaternion.identity);
yield return new WaitForSeconds(waveConfig.GetTimeBetweenSpawns());
}
}
Any help on sorting the double enemy at the start of the wave would be greatly appciated.
On a slightly different note on the
for (int enemyCount = 0;
enemyCount < waveConfig.GetNumberOfEnemies();
enemyCount++)
Why does it work with enemyCount < waveConfig.GetNumberOfEnemies() and not enemyCount <= waveConfig.GetNumberOfEnemies()? I thought it would be the <= and that is how I did it until I saw how Rick did it.
Thank you in advance!!