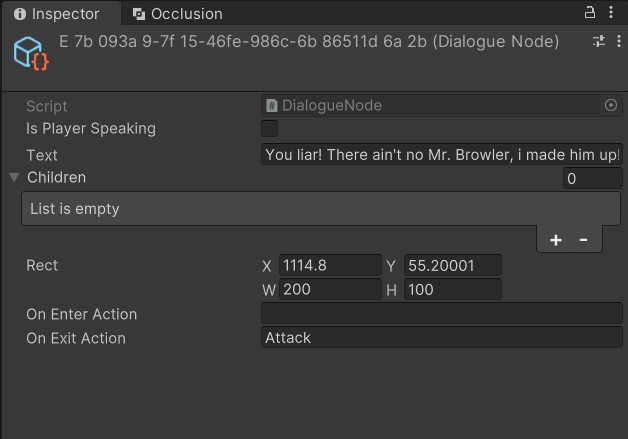
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using UnityEngine;
namespace RPG.Dialogue
{
public class PlayerConversant : MonoBehaviour
{
Dialogue currentDialogue;
DialogueNode currentNode = null;
AIConversant currentConversant = null;
bool isChoosing = false;
public event Action onConversationUpdate;
public void StartDialogue(AIConversant newConversant, Dialogue newDialogue)
{
currentConversant = newConversant;
currentDialogue = newDialogue;
currentNode = currentDialogue.GetRootNode();
TriggerEnterAction();
onConversationUpdate();
}
public void Quit()
{
currentDialogue = null;
TriggerExitAction();
currentNode = null;
isChoosing = false;
currentConversant = null;
onConversationUpdate();
}
public bool IsActive()
{
return currentNode != null;
}
public bool IsChoosing()
{
return isChoosing;
}
public string GetText()
{
if (currentNode == null)
{
return "";
}
return currentNode.GetText();
}
public IEnumerable<DialogueNode> GetChoices()
{
return currentDialogue.GetPlayerChildren(currentNode);
}
public void SelectChoice(DialogueNode chosenNode)
{
currentNode = chosenNode;
TriggerEnterAction();
isChoosing = false;
Next();
}
public void Next()
{
int numPlayerResponses = currentDialogue.GetPlayerChildren(currentNode).Count();
if (numPlayerResponses > 0)
{
isChoosing = true;
TriggerExitAction();
onConversationUpdate();
return;
}
DialogueNode[] children = currentDialogue.GetAIChildren(currentNode).ToArray();
int randomIndex = UnityEngine.Random.Range(0, children.Count());
TriggerExitAction();
currentNode = children[0];
TriggerEnterAction();
onConversationUpdate();
}
public bool HasNext()
{
return currentDialogue.GetAllChildren(currentNode).Count() > 0;
}
private void TriggerEnterAction()
{
if (currentNode != null)
{
Debug.Log($"Triggering action {currentNode.GetOnEnterAction()}");
TriggerAction(currentNode.GetOnEnterAction());
}
}
private void TriggerExitAction()
{
if (currentNode != null)
{
Debug.Log($"Triggering action {currentNode.GetOnExitAction()}");
TriggerAction(currentNode.GetOnExitAction());
}
}
private void TriggerAction(string action)
{
if (action == "") { Debug.Log("No Action"); return; }
foreach (DialogueTrigger trigger in currentConversant.GetComponents<DialogueTrigger>())
{
Debug.Log("Testing trigger");
trigger.Trigger(action);
}
}
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Events;
namespace RPG.Dialogue
{
public class DialogueTrigger : MonoBehaviour
{
[SerializeField] string action;
[SerializeField] UnityEvent onTrigger;
public void Trigger(string actionToTrigger)
{
Debug.Log($"{name} is testing trigger {actionToTrigger}. This trigger = {action}.");
if (actionToTrigger == action)
{
Debug.Log($"{actionToTrigger}=={action}. Condition matches");
onTrigger?.Invoke();
}
}
}
}
using RPG.Control;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
namespace RPG.Dialogue
{
public class AIConversant : MonoBehaviour, IRaycastable
{
[SerializeField] Dialogue dialogue = null;
public CursorType GetCursorType()
{
return CursorType.Dialogue;
}
public bool HandleRaycast(PlayerController callingController)
{
if (dialogue == null)
{
return false;
}
if (Input.GetMouseButtonDown(0))
{
callingController.GetComponent<PlayerConversant>().StartDialogue(this, dialogue);
}
return true;
}
}
}
I disable the fighter script and remove combat target. When i ended the conversation, fighter script is enable again but the enem doesn't move.