Hi, so under this lecture there is already a thread with the title “Character wont face the same direction as the camera”. This point got resolved, but I have the same problem and the solution there doesn’t apply to me. Can somebody help me? I’ll include screenshots of my code.
Hey @BlenderGuy,
- What exactly is happening?
- Do you get any errors in the console?
- Did you double-check your Cinemacine settings?
- Did you double-check your Prefab hierarchy / apply any overrides you have?
- Are you getting the correct camera values in PlayerStateMachine?
private void Start()
{
if (Camera.main != null) MainCameraTransform = Camera.main.transform;
}
You can post the code using the code tag in the editor - it’s the </> symbol. It’s easier to copy-paste it for debugging. Looking over the SS you posted, I don’t see anything wrong there.
Hi, thanks for the quick response. I checked again and I found that the problem actually is that the character doesn’t rotate in the direction the camera is looking. I couldn’t differnciate that before. I will send you my code again, maybe you will find something with this info or tell me where I have to search.
Also I have all overrides applied and there are no errors in the console.
`using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerFreeLookState : PlayerBaseState
{
private readonly int FreeLookSpeedHash = Animator.StringToHash("FreeLookSpeed");
private const float AnimatorDampTime = 0.1f;
public PlayerFreeLookState(PlayerStateMachine StateMachine) : base(StateMachine)
{
}
public override void Enter()
{
}
public override void Tick(float deltaTime)
{
Vector3 movement = CalculateMovement();
StateMachine.Controller.Move(movement * StateMachine.FreeLookMovementSpeed * deltaTime);
if (StateMachine.InputReader.MovementValue == Vector2.zero)
{
StateMachine.Animator.SetFloat(FreeLookSpeedHash, 0, AnimatorDampTime, deltaTime);
return;
}
StateMachine.Animator.SetFloat(FreeLookSpeedHash, 1, AnimatorDampTime, deltaTime);
FaceMovementDirection(movement, deltaTime);
}
public override void Exit()
{
}
private Vector3 CalculateMovement()
{
Vector3 forward = StateMachine.MainCameraTransfrom.forward;
Vector3 right = StateMachine.MainCameraTransfrom.right;
forward.y = 0f;
right.y = 0f;
forward.Normalize();
right.Normalize();
return forward * StateMachine.InputReader.MovementValue.y +
right * StateMachine.InputReader.MovementValue.x;
}
private void FaceMovementDirection(Vector3 movement, float deltaTime)
{
StateMachine.transform.rotation = Quaternion.Lerp(
StateMachine.transform.rotation,
Quaternion.LookRotation(movement),
deltaTime * StateMachine.RotationDamping
);
}
}
`
Hey @BlenderGuy, can you also post the code from PlayerStateMachine.cs.
Can you also post a screenshot of your Hierarchy and prefab structure? (like this one)
As a general rule to troubleshoot things, you can use the debug logs to see what values you get and where the problem is. For example
You can put a debug log in CalculateMovement() to see if you get any values from the camera
private Vector3 CalculateMovement()
{
Vector3 forward = StateMachine.MainCameraTransfrom.forward;
Vector3 right = StateMachine.MainCameraTransfrom.right;
forward.y = 0f;
right.y = 0f;
forward.Normalize();
right.Normalize();
Debug.Log($"Forward is {forward.ToString()} + Right is {right.ToString()}");
return forward * StateMachine.InputReader.MovementValue.y + right * StateMachine.InputReader.MovementValue.x;
}
Hi, so I tried the Debug.Log, and I’m getting values for the rotation of the camera, like in the Screenshot.
Maybe I have duck sth off in the Animation?
Anyways here is the prefab Hierarchy and StateMachine. Thanks for you help.
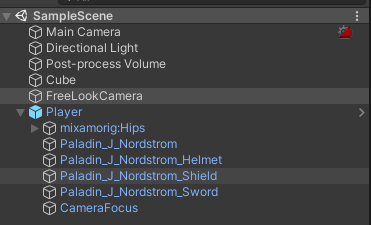
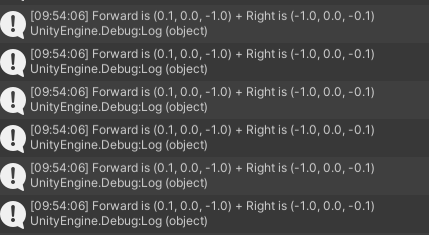
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerStateMachine : StateMachine
{
[field: SerializeField]public InputReader InputReader{get;private set;}
[field: SerializeField]public CharacterController Controller{get;private set;}
[field: SerializeField]public float FreeLookMovementSpeed{get;private set;}
[field: SerializeField]public float RotationDamping{get;private set;}
[field: SerializeField]public Animator Animator{get;private set;}
public Transform MainCameraTransfrom{get;private set;}
private void Start()
{
MainCameraTransfrom = Camera.main.transform;
SwitchState(new PlayerFreeLookState(this));
}
}
Hmm, they all look good to me. When you have the time, please do the following
- Create a new test scene with just the player/ground/camera and test it there. It it’s still broken, do the following .
- Right-click the Test Scene file and click “Select Dependencies”
- Right-click again and select “Export-Package”
- Upload that package to Dropbox/Google Drive/ Etc and paste the link here so I can download it and take a better look.
Here comes my Dropboxlink. And again thank you very much for the help.
It works, thank you kindly for your efforts.
This topic was automatically closed 24 hours after the last reply. New replies are no longer allowed.