I’m currently stumped. I have a quest that works like this: A guard has a question, and I have to ask the general that question for him. While asking the question, I have 3 routes on how I want to approach it. A good, bad, and neutral route. The neutral route includes the general giving me a note to give to the guard. The other two are reliant on the player’s dialogue decisions. After I return to the guard, what I tell him at that point is reliant on what I had said to the general.
I had a working system where I just added a function called ChangeCurrentDialogue() to the DialogueTrigger script and it worked great for that, because I could have multiple different dialogues on each character for what ends up happening, but it got to be a lot of DialogueTrigger components on each character, so I figured these predicates would be the solution to that problem. I have not been able to figure out if there’s a way on how I could somehow link these triggers and predicates together, and just have one big dialogue with different responses to the one node, as opposed to having a bunch of different dialogues for each possible outcome. How could this be done, exactly? And would this even be an ideal thing to do, or is there a better way?
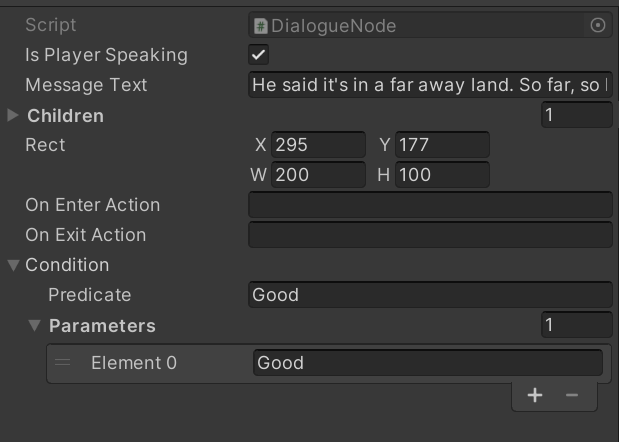
I just simply added a serializefield to the dialogueTriggerHolder, and set it to be the general’s GameObject: