When I click play my player character doesn’t approach the enemy, instead I get this error. I’ve gone over the course a few times by now and have triple checked everything. I am hoping I am just overseeing something small that someone can help me with. Thank you for your time.
In Awake(), you need to set navMeshAgent. Should look something like this:
private void Awake()
{
//...you may already have other code here
navMeshAgent = GetComponent<NavMeshAgent>(); //add this line
}
Once you set that, it should fix your problem, and you can optimize some of your other code since you will have a cached reference to the NavMeshAgent component. For example:
public void MoveTo(Vector3 destination)
{
navMeshAgent.destination = destination;
navMeshAgent.isStopped = false;
}
and in UpdateAnimator() you can modify line 62:
Vector3 velocity = navMeshAgent.velocity;
Remember that GetComponent() is a fairly expensive operation to call every frame. Caching the NavMeshAgent in the navMeshAgent variable and using that is the way to go. Hope that helps.
Thank you for the reply but I’m just getting different issues now. I’m not getting error messages now but clicking on the enemy doesn’t do anything.
Can you post the full mover script please? Does clicking on the ground work? The issue could be in PlayerController.cs if click-to-move works when you click on the ground, but not when you click an enemy.
I’ve been working on this all night so if things look crazy right now I don’t doubt it. But this is where I am at so far. Thank you for your help.
Have you attached the combat target script to the enemy prefab or just one in the scene and forgot to apply to the prefab.
Ok, just to confirm, your click-to-move is working, but click-to-attack (when you click on enemy) does nothing, correct? If so, the next thing to check is your Warrior.cs Post that when you get a chance, please. Also, let’s try a little test. Inside PlayerController -> InteractWithCombat() , around line 28:
--------
if (Input.GetMouseButtonDown(0)
{
print("clicked on enemy"); //add this line
GetComponent<Warrior>().Attack(target);
}
---------
After adding the print statement, does the “clicked on enemy” message appear in the console when you click on an enemy?
Well that does show that I am clicking on the enemy. It’s just that nothing happens. clicking on the ground works and I can move my player, but when I click the enemy he just stands there.
Also here is my warrior(fighter) script.
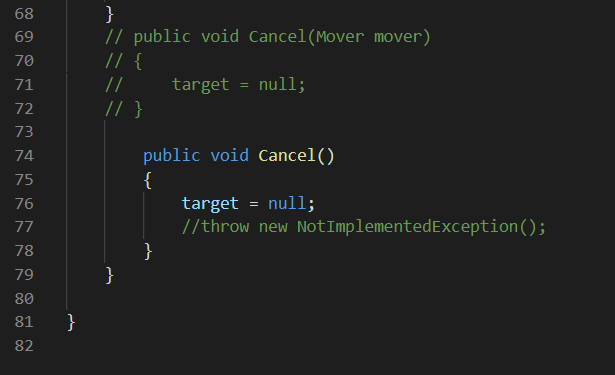
In Warrior.cs try this:
public void Attack(CombatTarget combattarget)
{
GetComponent<ActionScheduler>().StartAction(this);
target = combattarget.transform;
GetComponent<Mover>().MoveTo(target.position); //add this line
}
That helps, now when I click the enemy my player moves to the enemy and it does show I clicked the enemy.
Thought I had it figured out, went back through all the videos and redone all the code just to make sure I got everything correct and now when I press play I’m getting this.
Im up to Section 4 Basic Combat, Video 36. I stopped here because of this bug and I keep going back through the videos and checking and I am not seeing why when I press play its different than the video in the course.
In Warrior.cs – > GetIsInRange(), you need to check if you have a target, like so:
private bool GetIsInRange()
{
if (target == null) return false; //add this check
return Vector3.Distance(transform.position, target.position) < weaponRange;
}
Need to make sure target is not null: change line 25 to:
if (!GetIsInRange() && target != null)
I don’t suppose the code to the lesson is somewhere I can download it so I can go between their code and mine to see whats going on?
Did you remove this line from your Mover.cs script? It assigns a value to navMeshAgent and would prevent that null reference exception.
private void Awake()
{
//...you may already have other code here
navMeshAgent = GetComponent<NavMeshAgent>(); //add this line
}
Then the other thing to check is that every object that has a Mover script attached also has a NavMeshAgent component on it. If it doesn’t have one, Start() can’t find it to assign it.