I wrote all the code out and tried to test the scoreboard but it said “Not set to an instance of an object.” My text component looks slightly different than in the video. I’m not sure if it still has the same reference name.
Can you show the error, then we’ll work from there
Hi Jaden1,
To which game object is the Scoreboard component attached?
In line 12, the code looks for a Text object. In your first screenshot, we can see a TextMeshProUGUI component, not a Text component. Depending on what type of component is supposed to be referenced in your code, you’ll have to adjust the code.
Have you already compared your code to the Lecture Project Changes which can be found in the Resources of this lecture?
See also:
- Forum User Guides : How to apply code formatting within your post
- Forum User Guides : How to mark a topic as solved
The problem is that you are using TextMeshPro, but looking for an old Unity text field. You should be looking for a TextMeshPro field
scoreText = GetComponent<TMPro.TextMeshProUGUI>();
Edit Oh, @Nina already pointed this out…
using UnityEngine.UI;
using UnityEngine;
using TMPro;
public class Scoreboard : MonoBehaviour
{
int score;
Text scoreText;
void Start()
{
score = 0;
scoreText = GetComponent<TMPro.TextMeshProUGUI>();
scoreText.text = score.ToString();
}
// Update is called once per frame
void Update()
{
}
}
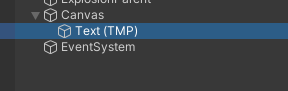
The second picture is where I placed the script. It still has an error. I did not have a way to just text. The only option was “Text(TMP).”
This is because you have still defined the scoreText
as Text
using UnityEngine.UI;
using UnityEngine;
using TMPro;
public class Scoreboard : MonoBehaviour
{
int score;
TextMeshProUGUI scoreText; // <-- you need to define this correctly
void Start()
{
score = 0;
scoreText = GetComponent<TextMeshProUGUI>(); // <-- you don't need TMPro here because you defined it as a 'using' at the top
scoreText.text = score.ToString();
}
// Update is called once per frame
void Update()
{
}
}
Ohhhh that makes a lot of sense now. Thank you!
If you get the ‘Cannot implicitly convert type’ error, always check if your object type matches the target type. Here, the object type would be TextMeshProUGUI in the GetComponent method, which returns an object (or no object: null
), and the target type would be the data type of the scoreText
variable. We usually cannot assign objects to a variable of a different type.
There are data types that can be converted implicitely but in this course we do not convert anything, so don’t worry if you found that conversion thing confusing. If you follow the aforementioned rule, you will be able to avoid or fix that error yourself.
This topic was automatically closed 24 hours after the last reply. New replies are no longer allowed.