When I create a child node and undo it, the child node disappears but the children’s unique ID list doesn’t delete the child node’s uid. Also, the connection still stays because of this, but there’s no node to connect.
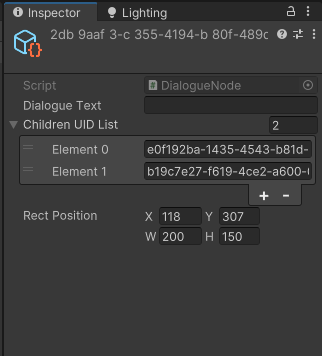
I’ve compared the code from this lecture’s commit and they’re more or less the same.
I’m guessing that the undo operation before node creation: Undo.RegisterCreatedObjectUndo deletes the created node but doesn’t update the list.
I was thinking of using something like Undo.undoRedoEvent (which gives us info about the undo group and name) to remove the child node from the Dialogue children list. But I’m not sure how to get the data of the child node after performing the Undo operation.