For some reason, my sprites aren’t updating when hit by the ball. They are destrying as per the number of hits in the serialized field but the sprites just are not updating.
public class Block : MonoBehaviour
{
//config params
[SerializeField] AudioClip breakSound;
[SerializeField] GameObject blockSparklesVFX;
[SerializeField] int maxHits;
[SerializeField] Sprite hitSprites;
//cached reference
Level level;
//state variables
[SerializeField] int timesHit; //TODO only serialized for debug purposes
private void Start()
{
CountBreakableBlocks();
}
private void CountBreakableBlocks()
{
level = FindObjectOfType<Level>();
if (tag == "Breakable")
{
level.CountBlocks();
}
}
private void OnCollisionEnter2D(Collision2D collision)
{
HandleHit();
}
private void HandleHit()
{
if (tag == "Breakable")
{
timesHit++;
if (timesHit >= maxHits)
{
DestroyBlock();
}
}else
{
ShowNextHitSprite();
}
}
private void ShowNextHitSprite()
{
int spriteIndex = timesHit - 1;
GetComponent<SpriteRenderer>().sprite = hitSprites[spriteIndex];
}
private void DestroyBlock()
{
PlayBlockDestroySFX();
level.BlockDestroyed();
Destroy(gameObject);
TriggerSparklesVFX();
}
private void PlayBlockDestroySFX()
{
FindObjectOfType<GameSession>().AddToScore();
AudioSource.PlayClipAtPoint(breakSound, Camera.main.transform.position);
}
private void TriggerSparklesVFX()
{
GameObject sparkles = Instantiate(blockSparklesVFX, transform.position, transform.rotation);
Destroy(sparkles, 1f);
}
}
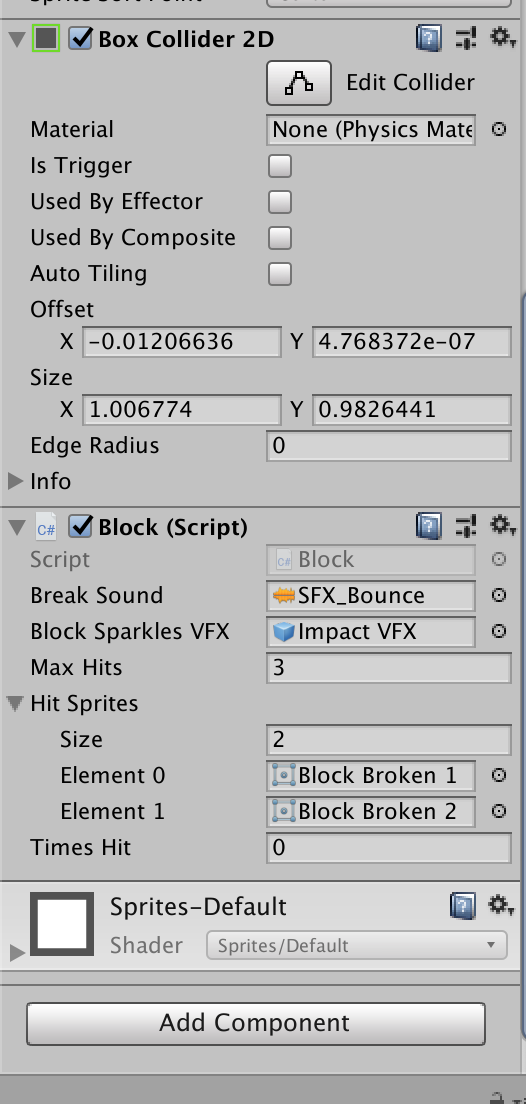