I get the message saying that I got the wrong answer even if I choose the right one, everything else works great.
I’ve checked the code and settings and it all seems fine but maybe I am missing something?
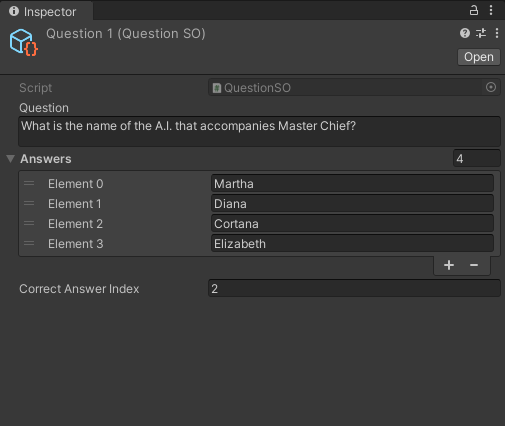
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using TMPro;
public class Quiz : MonoBehaviour
{
[SerializeField] TextMeshProUGUI questionText;
[SerializeField] QuestionSO question;
[SerializeField] GameObject[] answerButtons;
int correctAnswerIndex;
[SerializeField] Sprite defaultAnswerSprite;
[SerializeField] Sprite correctAnswerSprite;
void Start()
{
questionText.text = question.GetQuestion();
for (int i = 0; i < answerButtons.Length; i++)
{
TextMeshProUGUI buttonText = answerButtons[i].GetComponentInChildren<TextMeshProUGUI>();
buttonText.text = question.GetAnswer(i);
}
}
public void OnAnswerSelected(int index)
{
Image buttonImage;
if (index == question.GetCorrectAnswerIndex())
{
questionText.text = "Correct!";
buttonImage = answerButtons[index].GetComponent<Image>();
buttonImage.sprite = correctAnswerSprite;
}
else
{
correctAnswerIndex = question.GetCorrectAnswerIndex();
string correctAnswer = question.GetAnswer(correctAnswerIndex);
questionText.text = "Sorry, the correct answer was; \n" + correctAnswer;
buttonImage = answerButtons[correctAnswerIndex].GetComponent<Image>();
buttonImage.sprite = correctAnswerSprite;
}
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[CreateAssetMenu(menuName = "Quiz Question", fileName = "New Question")]
public class QuestionSO : ScriptableObject
{
[TextArea(2,6)]
[SerializeField] string question = "Insert question here";
[SerializeField] string[] answers = new string[4];
[SerializeField] int correctAnswerIndex;
public string GetQuestion()
{
return question;
}
public string GetAnswer(int index)
{
return answers[index];
}
public int GetCorrectAnswerIndex()
{
return correctAnswerIndex;
}
}```