Hey guys!
Carl is correct - you are crashing because you are trying to call a method on a pointer to an object that doesn’t exist.
The first issue I spotted with the code is that ATriggerValue* PressurePlate
needs to have the UPROPERTY(EditAnywhere)
macro on the line above it. This allows you assign a reference to the object from the editor itself.
Next, you probably just need to set the reference to the pressure plate in your world from the Door_BP details panel. Ben covers this in lecture 72 around the 7:45 mark, but here’s what you need to set:
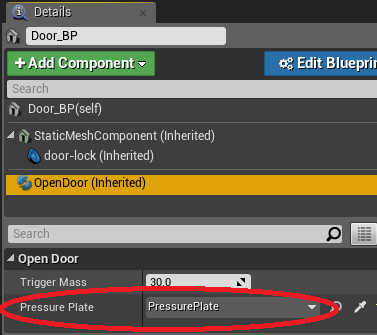
On a side note, generally something in the form of <type> VariableName
is referred to as a declaration, and setting the variable to a value is an assignment. You could argue that something like int x = 0
is a declaration, a definition, and an assignment all in one statement (msdn has a pretty good explanation: https://msdn.microsoft.com/en-us/library/0e5kx78b.aspx). Also if you right-click the variable PressurePlate
and select either “Go To Definition” or “Go To Declaration” it will take you to the same place. However, this is not the case with functions (try the same context menu options on a function to see what I mean).
Anyway, the statement ATriggerVolume* PressurePlate
just declares a pointer to ATriggerVolume named PressurePlate, whereas a line like PressurePlate = new ATriggerVolume;
actually creates a new instance of ATriggerVolume and assigns it to PressurePlate (C++11 people don’t shoot me, it’s just an example for simplicity sake ;)). However, in our case, we’ve already created an instance of the PressurePlate in our world, so we just need to assign it in the editor as shown above.
Lastly, it’s always good to check pointers for validity before calling a method on them i.e. if (PressurePlate != nullptr) { // Do stuff with PressurePlate... }
which can be simplified to if (PressurePlate) { // ... }
.
Hope that helps!