You can’t really do it easily, but there are ways;
one (quite simple) way would be to add a flag to the units that you can set in the inspector. Then, you can add a small check in the code for this flag and put the breakpoint in the check. Unfortunately, you’d have to have the code ready at design time because you can’t edit it at runtime
This is the flag
[SerializeField] bool debugUnit;
Now, in the inspector you can find the unit and check the box in the inspector. In the spot you want the breakpoint, add this
if (debugUnit)
{ // put the breakpoint on this line
}
Now the debugger will only break in the unit where the breakpoint is set and you can step through that. You don’t need code in the if
, just the braces so you can put the breakpoint on the brace. The code will only hit the brace if the flag is set, so it will only hit the breakpoint then
Note: the next bit works in Visual Studio. I don’t know if it will work in VSCode
Another way is to figure out (at runtime) what the unit’s unique id is. You could use the hash code of the instance to determine this. Then, you can set a condition on the breakpoint to check the hash code and it will only break if the condition is met.
You could also use the debugUnit
flag above with conditions, but conditions slows the code down tremendously.
To set a condition on a breakpoint, right-click on the dot and pick Conditions...
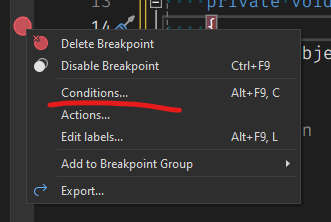
For the flag, the condition would be something like debugUnit == true
One other thing is that you could probably get away with not using the debugger. It depends on what you are trying to check. In most cases you could just write to the console. For this, I’d use the debugUnit
and then just write the data if the flag is set
if (debugUnit)
{
Debug.Log(DataImInterestedIn);
}
In my day job I debug a lot, but with Unity I seldom do. I’ve found Unity to start slowing down the longer I debug so I’ve learned to get what I need without running the debugger.