Hello Duncan,
Welcome to the community 
You have a few things to deal with here.
Firstly, you are using a version of Unity which is more recent than that which the course was created using, there have been numerous API changes in that period of time. Thankfully, the ones that are relevant to the course are documented in a Google Doc which you can find linked under Resources on the Udemy website.
Specifically though, for this issue, you will need to do the following;
Add this line to the top of your script;
using UnityEngine.SceneManagement;
Next, within your LoadLevel
method, replace this line;
Application.LoadLevel(name);
with the following;
SceneManager.LoadScene(name);
That takes care of the differences due to the API changes so far.
Your error about the Build Settings could be due to not specifying the name of the scene you wanted to load in your screenshot above, but we should check that you have your scene actually in your Build Settings, as that will also generate this error.
So, within Unity, goto File -> Build Settings
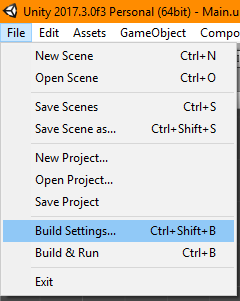
On the top you can see a pane which will display all of the scenes which you have added to be a part of your project;
If your scene is not currently listed, you can either use the Add Open Scenes button, or just drag it from the Assets folder into this pane.
Also, you can just copy/paste your code directly into the forum, this is often more useful than a screenshot as it allows those who offer to help you the ability to copy/paste from your code instead of having to type it all in themselves. Screenshots are however very useful when it comes to error messages or details from within the Unity editor itself.
Hope this helps. 
See also;