You would have to take Brian’s solution and dumb it down a bit.
First, you will need somewhere to keep track of all enemies that are killed. You can simply add a script to the player that holds this info, and add a way for the enemies to update this script (using the OnDie
event of the Health
component)
On the player, we can keep the death counter
public class DeathCounter : MonoBehaviour
{
private Dictionary<string, int> counterDict = new Dictionary<string, int>();
public void RegisterCounter(string victim)
{
if (!counterDict.ContainsKey(victim))
{
counterDict.Add(victim, 0);
}
}
public void RecordDeath(string victim)
{
if (!counterDict.ContainsKey(victim))
{
return;
}
counterDict[victim]++;
}
public int GetDeathCount(string victim)
{
if (!counterDict.ContainsKey(victim)) return 0;
return counterDict[victim];
}
}
On the enemies, we need to add the death recorder
public class DeathRecorder : MonoBehaviour
{
[SerializeField] string enemyIdentifier;
public void RecordDeath()
{
var counter = GameObject.FindWithTag("Player").GetComponent<DeathCounter>();
counter.RecordDeath(enemyIdentifier);
}
}
And this goes on the enemies
Now, create a script that could be used to check the kills (I have no safety checks in here)
public class KillEvaluator : MonoBehaviour, IPredicateEvaluator
{
[SerializeField] DeathCounter deathCounter;
public bool? Evaluate(string predicate, string[] parameters)
{
if (predicate != "Has Killed") return null;
var victim = parameters[0];
var requiredKills = int.Parse(parameters[1]);
deathCounter.RegisterCounter(victim);
return deathCounter.GetDeathCount(victim) >= requiredKills;
}
}
Put this, and the DeathCounter
script on the player
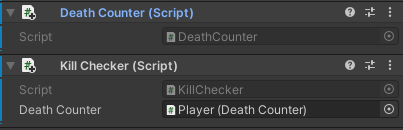
and use it in your dialogues (My predicates are scriptable objects, but you get the gist)
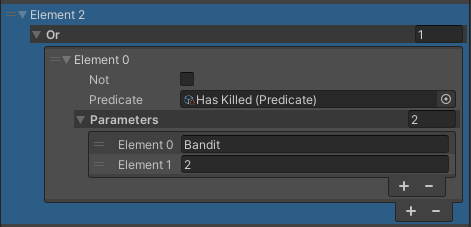
NOTE I haven’t tested this properly yet, and my own dialogues are broken at the moment. Busy sorting it out so I can test this