I’ve added a c++ component, but I can’t see it through Add. Why?
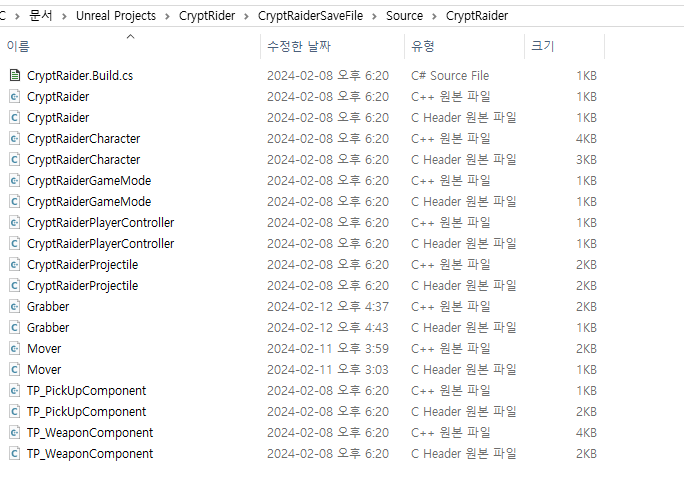
Both grabber and mover belong to the Unreal Engine folder I ran. Why isn’t it coming out? It came out well at first, but it started not coming out at some point.
I’ve added a c++ component, but I can’t see it through Add. Why?
Both grabber and mover belong to the Unreal Engine folder I ran. Why isn’t it coming out? It came out well at first, but it started not coming out at some point.
Could you show the Mover files please?
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "Components/ActorComponent.h"
#include "Math/UnrealMathUtility.h"
#include "Mover.generated.h"
UCLASS( ClassGroup=(Custom), meta=(BlueprintSpawnableComponent) )
class CRYPTRAIDER_API UMover : public UActorComponent
{
GENERATED_BODY()
public:
// Sets default values for this component's properties
UMover();
protected:
// Called when the game starts
virtual void BeginPlay() override;
public:
// Called every frame
virtual void TickComponent(float DeltaTime, ELevelTick TickType, FActorComponentTickFunction* ThisTickFunction) override;
private:
UPROPERTY(EditAnywhere)
FVector MoveOffset;
UPROPERTY(EditAnywhere)
float MoveTime = 4;
UPROPERTY(EditAnywhere)
bool ShouldMove = false;
FVector OriginalLocation;
};
#include "Mover.h"
#include "Math/UnrealMathUtility.h"
// Sets default values for this component's properties
UMover::UMover()
{
// Set this component to be initialized when the game starts, and to be ticked every frame. You can turn these features
// off to improve performance if you don't need them.
PrimaryComponentTick.bCanEverTick = true;
// ...
}
// Called when the game starts
void UMover::BeginPlay()
{
Super::BeginPlay();
OriginalLocation = GetOwner()->GetActorLocation();
// ...
}
//컴포넌트를 통해 액터에게 사운드를 부여하거나 액터의 위치를 파악하거나 설정하는 등의 작업을 수행할려면 포인터를 액터에게 먼저 전달해야한다.
// Called every frame
void UMover::TickComponent(float DeltaTime, ELevelTick TickType, FActorComponentTickFunction* ThisTickFunction)
{
Super::TickComponent(DeltaTime, TickType, ThisTickFunction);
if (ShouldMove){
FVector CurrentLocation = GetOwner()->GetActorLocation();
FVector TargetLocation = OriginalLocation + MoveOffset;
float Speed = FVector::Distance(OriginalLocation, TargetLocation) / MoveTime;
FVector NewLocation = FMath::VInterpConstantTo(CurrentLocation, TargetLocation, DeltaTime, Speed);
GetOwner() -> SetActorLocation(NewLocation);
}
}
Did you compile successfully? Could you show the output of compilation?
Registered process C:\Program Files\Epic Games\UE_5.3\Engine\Binaries\Win64\UnrealEditor.exe (PID: 6756)
Loading module C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Binaries\Win64\UnrealEditor-CryptRaider.dll (0.283 MB)
Loaded 1 module(s), 673 lazy load module(s), and 424 reserved page ranges (0.000s, 15 translation units)
Live coding ready - Save changes and press Ctrl+Alt+F11 to re-compile code
Manual recompile triggered
---------- Creating patch ----------
Running C:\Program Files\Epic Games\UE_5.3\Engine\Build\BatchFiles\Build.bat -Target=“CryptRaiderEditor Win64 Development -Project=”“C:/Users/82103/Documents/Unreal Projects/CryptRider/CryptRaiderSaveFile/CryptRaider.uproject”"" -LiveCoding -LiveCodingModules=“C:/Program Files/Epic Games/UE_5.3/Engine/Intermediate/LiveCodingModules.json” -LiveCodingManifest=“C:/Program Files/Epic Games/UE_5.3/Engine/Intermediate/LiveCoding.json” -WaitMutex -LiveCodingLimit=100
Quick restart disabled when re-instancing is enabled.
Using bundled DotNet SDK version: 6.0.302
Running UnrealBuildTool: dotnet “…\Engine\Binaries\DotNET\UnrealBuildTool\UnrealBuildTool.dll” -Target=“CryptRaiderEditor Win64 Development -Project=”“C:/Users/82103/Documents/Unreal Projects/CryptRider/CryptRaiderSaveFile/CryptRaider.uproject”"" -LiveCoding -LiveCodingModules=“C:/Program Files/Epic Games/UE_5.3/Engine/Intermediate/LiveCodingModules.json” -LiveCodingManifest=“C:/Program Files/Epic Games/UE_5.3/Engine/Intermediate/LiveCoding.json” -WaitMutex -LiveCodingLimit=100
Log file: C:\Users\82103\AppData\Local\UnrealBuildTool\Log.txt
Invalidating makefile for CryptRaiderEditor (source file removed)
Parsing headers for CryptRaiderEditor
Running Internal UnrealHeaderTool “C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\CryptRaider.uproject” “C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\CryptRaiderEditor\Development\CryptRaiderEditor.uhtmanifest” -WarningsAsErrors -installed
Total of 1 written
Reflection code generated for CryptRaiderEditor in 4.007374 seconds
Building CryptRaiderEditor…
Using Visual Studio 2022 14.38.33135 toolchain (C:\Program Files\Microsoft Visual Studio\2022\Community\VC\Tools\MSVC\14.38.33130) and Windows 10.0.22621.0 SDK (C:\Program Files (x86)\Windows Kits\10).
Determining max actions to execute in parallel (6 physical cores, 12 logical cores)
Executing up to 6 processes, one per physical core
------ Building 19 action(s) started ------
[1/19] Compile [x64] SharedPCH.Engine.Cpp20.cpp
[2/19] Compile [x64] CryptRaider.cpp
[3/19] Compile [x64] CryptRaiderPlayerController.gen.cpp
[4/19] Compile [x64] Grabber.cpp
[5/19] Compile [x64] CryptRaider.init.gen.cpp
[6/19] Compile [x64] CryptRaiderGameMode.gen.cpp
[7/19] Compile [x64] TP_PickUpComponent.gen.cpp
[8/19] Compile [x64] TP_WeaponComponent.gen.cpp
[9/19] Compile [x64] TP_PickUpComponent.cpp
[10/19] Compile [x64] Grabber.gen.cpp
[11/19] Compile [x64] CryptRaiderPlayerController.cpp
[12/19] Compile [x64] Mover.cpp
[13/19] Compile [x64] Mover.gen.cpp
[14/19] Compile [x64] CryptRaiderCharacter.cpp
[15/19] Compile [x64] CryptRaiderProjectile.gen.cpp
[16/19] Compile [x64] CryptRaiderGameMode.cpp
[17/19] Compile [x64] CryptRaiderCharacter.gen.cpp
[18/19] Compile [x64] CryptRaiderProjectile.cpp
[19/19] Compile [x64] TP_WeaponComponent.cpp
Total time in Parallel executor: 21.23 seconds
Total execution time: 27.15 seconds
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\CryptRaiderEditor\Development\Engine\SharedPCH.Engine.Cpp20.h.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\CryptRaider.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\CryptRaider.init.gen.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\CryptRaiderCharacter.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\CryptRaiderCharacter.gen.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\CryptRaiderGameMode.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\CryptRaiderGameMode.gen.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\CryptRaiderPlayerController.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\CryptRaiderPlayerController.gen.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\CryptRaiderProjectile.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\CryptRaiderProjectile.gen.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\Grabber.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\Grabber.gen.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\Mover.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\Mover.gen.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\TP_PickUpComponent.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\TP_PickUpComponent.gen.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\TP_WeaponComponent.cpp.obj was modified or is new
File C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Intermediate\Build\Win64\x64\UnrealEditor\Development\CryptRaider\TP_WeaponComponent.gen.cpp.obj was modified or is new
Building patch from 19 file(s) for Live coding module C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Binaries\Win64\UnrealEditor-CryptRaider.dll
C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaider\Binaries\Win64\UnrealEditor-CryptRaider.patch_0.lib 라이브러리 및 C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaider\Binaries\Win64\UnrealEditor-CryptRaider.patch_0.exp 개체를 생성하고 있습니다.
Successfully linked patch (0.000s)
When the compilation is complete, this message appears, but when it is finished, the unreal engine closes with an error. The error code is as follows.
LoginId:f28f92624aebe9c3444b2dbfe319b1d4
EpicAccountId:6f2ca09bbf054b96b98c090d58bdc220Fatal error: [File:D:\build++UE5\Sync\Engine\Source\Runtime\Core\Private\Logging\LogSuppressionInterface.cpp] [Line: 451] Log suppression category LogTemplateCharacter was somehow declared twice with the same data.
UnrealEditor_Core
UnrealEditor_Core
UnrealEditor_Core
UnrealEditor_CryptRaider_patch_0!`dynamic initializer for ‘LogTemplateCharacter’’() [C:\Users\82103\Documents\Unreal Projects\CryptRider\CryptRaiderSaveFile\Source\CryptRaider\CryptRaiderCharacter.cpp:14]
ucrtbase
UnrealEditor_CryptRaider_patch_0!dllmain_crt_process_attach() [D:\a_work\1\s\src\vctools\crt\vcstartup\src\startup\dll_dllmain.cpp:66]
UnrealEditor_CryptRaider_patch_0!dllmain_dispatch() [D:\a_work\1\s\src\vctools\crt\vcstartup\src\startup\dll_dllmain.cpp:276]
UnrealEditor_LiveCoding
UnrealEditor_LiveCoding
UnrealEditor_LiveCoding
ucrtbase
kernel32
ntdll
Could you show CryptRaiderCharacter.cpp and highlight line 14, please?
What files do you have in Public/Private?
Could you do a CTRL + F and search for LogTemplateCharacter and show the results?
Sorry, use Ctrl + Shift + F to search your whole workspace. The error says that log category is defined twice, so you have it defined once in that character.cpp and presumably somewhere else.
(You’ve also added that text to line 8 which you would need to remove)
I didn’t mean delete line 8, I meant delete the LogTemplateCharacter
bit you had at the end.
There doesn’t appear to be multiple definitions by the looks of. Have you tried a rebuild? You can do that by deleting the Binaries and Intermediate folders and then attempting to open the project.
Are you saying to delete LogTemplateCharacter after line 8? I will delete it.
In the screenshot I mentioned you had
#include "..."
#include "header.h"LogTemplateCharacter
#include "..."
....
I’m saying to remove that extraneous bit at the end.
Delete Binaries and Intermediate folders. I’ll try that, too
The issue has been resolved. Thank you so much. I’m curious about how this error occurred in addition, could you please let me know?
Usually when you build you only compile files that have changed and reuse previous compiled code for what didn’t. Deleting those folders deletes all compiled and generated code so you can build from scratch.
So it was likely a result from reusing an old compiled file which should have been recompiled.