There is actually another method, I just wasn’t thinking the other night when I posted the feet=zed method (my main project deals with a flat procedurally generated map, making the feet=zed ideal). This other method requires a bit of math, but fortunately, the Quaternion class does most of that nasty math for us…
Vector3 lookDirection = target.transform.position - transform.position;
lookDirection.y = 0;
Quaternion lookRotation = Quaternion.LookRotation(lookDirection, Vector3.up);
transform.rotation = lookRotation;
Here’s how this works… first, we calculate the vector from the target to the character. This is a normal mathematical vector, so much in the x direction, so much in the y, so much in the z. Once we have this vector, we zero out the y component, effectively flattening the map, at least that’s what we’re going to tell Quaternion…
So for our Obi Wan Kenobi vs. Annakin example in the illustration above (Always have the high ground!), the vector might be (-2, 3, 1). We’ll change that to (-2, 0, 1)
Now we’ll calculate the new Look Rotation passing this modified lookdirection in and setting the upwards vector to Vector3.up. Quaternion.LookRotation() does all the crazy math that turns -2,0,1 into the correction direction. We set that as the rotation and the character will stay standing upright.
Of course, this is going to look odd as well, as you can see with my unarmed Annakin punching obi-wan’s feet.
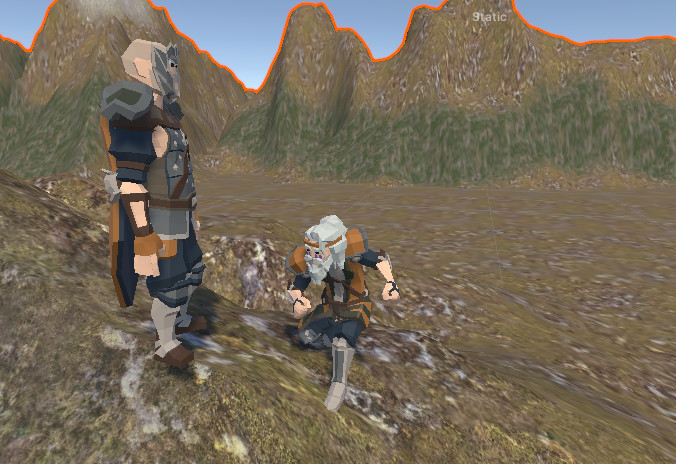
This can be fixed, by setting up IK chains and modifying the actual pose dynamically to make the fist or sword punch towards the center of the capsule, but… that’s a whole potential course in and of itself.