I have a problem with my score where I go from 750 to 300 to 450 to 600 750 900 and then 7050 …
my score display is:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ScoreDisplay : MonoBehaviour
{
Text scoreText;
GameSession gameSession;
// Use this for initialization
void Start()
{
scoreText = GetComponent<Text>();
gameSession = FindObjectOfType<GameSession>();
}
// Update is called once per frame
void Update()
{
scoreText.text = gameSession.GetScore().ToString();
}
}
my game session is:
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GameSession : MonoBehaviour
{
int score = 0;
private void Awake()
{
SetUpSingleton();
}
private void SetUpSingleton()
{
int numberGameSessions = FindObjectsOfType<GameSession>().Length;
if (numberGameSessions > 1)
{
Destroy(gameObject);
}
else
{
DontDestroyOnLoad(gameObject);
}
}
public int GetScore()
{
return score;
}
public void AddToScore(int scoreValue)
{
score += scoreValue;
}
public void ResetGame()
{
Destroy(gameObject);
}
}
I’ve called the score:
FindObjectOfType<GameSession>().AddToScore(scoreValue);
And … the score addes itself up, but not counting from 0 to 150’s
This is where I set the score to 150:
I can’t send I vidoe of the problem because it’s a mov. … However, I could send screenshots of the video
The first time I score it shows this:
2nd time:
3rd time:
4th time
5th time:
6th time:
7th time:
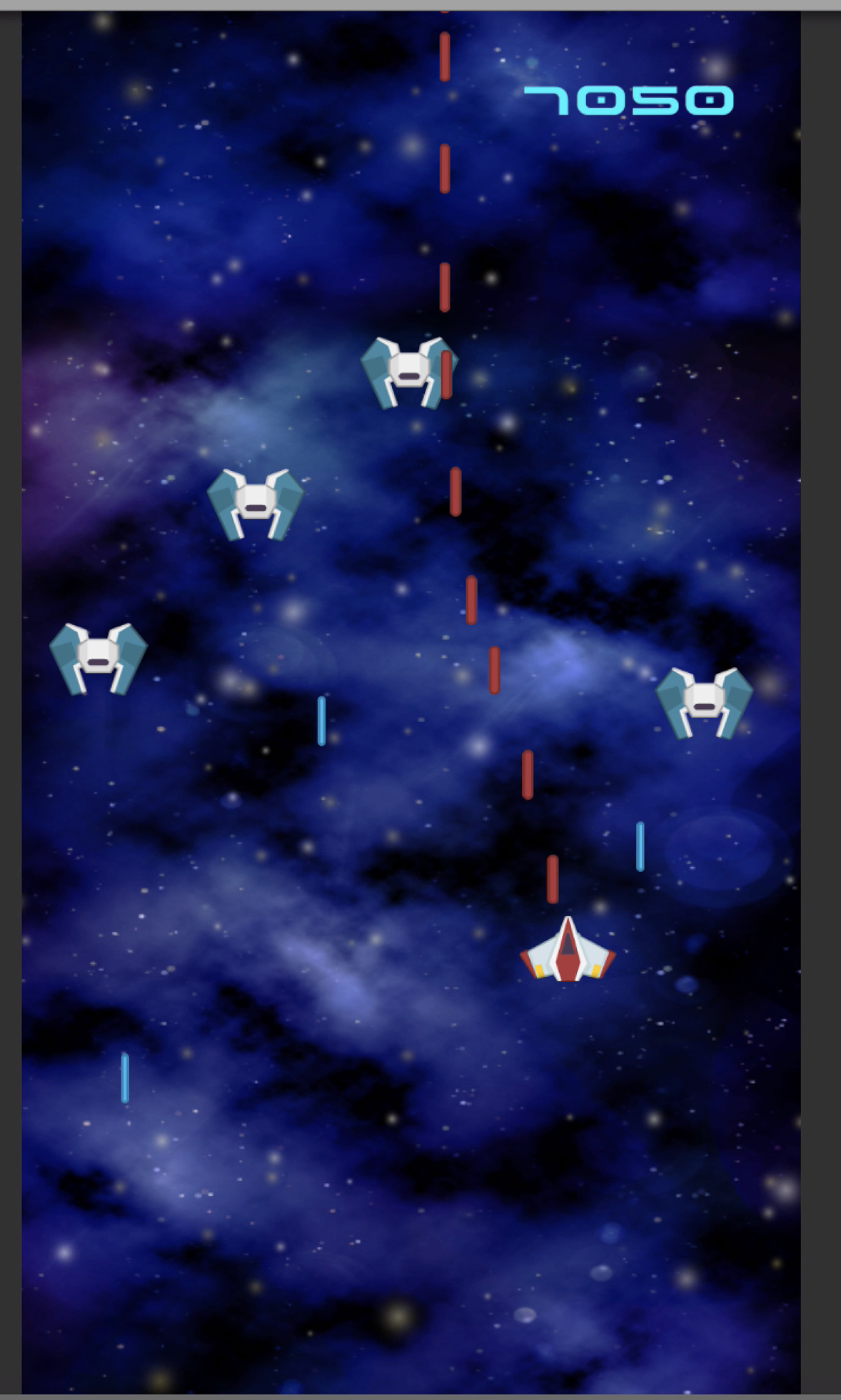
Really weird … and I haven’t got the slightest clue to why it’s happening. All my scripts are right. I attached them in the right places, so why is the score going up like crazy. I have nothing random in the scripts I have. So why is it like this why do I start at 750, and then go like normal untill I get passed 900 where I have 7050???