Let me give you an example, take a look at this:
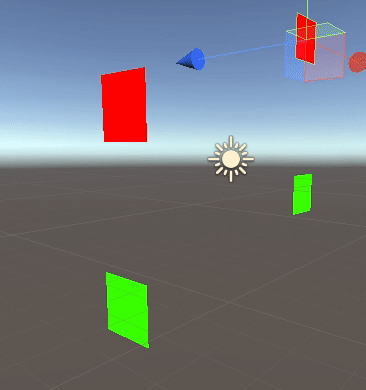
In the image above there are four cubes; the ones that are closer to the camera are rotated by 90 degrees on the Y axis, the ones further have a rotation of (0,0,0). The 2 red cubes have a Rigidbody2D and a collider, the green ones only a collider.
You can clearly see that if you rotate an object that is using 2D physics, the collisions will behave weirdly. That means that rotating the camera alongside the player will work to achieve the effect you are looking for, but also means that colliders will change in size. Also take a look at this other gif:
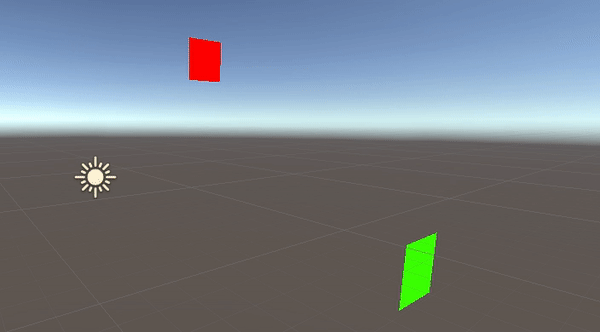
Here we have a very similar situation, the red square has a collider2D as so does the green square, you can see the red cube stops even tho they are not truly touching each other, at least not in the Z axis, that’s because the Z axis doesn’t exists in 2D, meaning they will collide regardless, again, that will cause issues with the rotation of the camera, the player will collide with enemies that aren’t even in sight.
You’ll have to figure out how to achive your effect taking this issues into consideration.