using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class NumberWizard : MonoBehaviour
{
int maxNumToGuess = 1000;
int minNumToGuess = 1;
int guess = 500;
void Start ()
{
Debug.Log("You know what Wizards are good at? GUESSING NUMBERS! True story.");
Debug.Log("I'm the Number Wizard. Pick a number, I'll try and guess what it is!");
Debug.Log("The highest number you can pick is: " + maxNumToGuess + ".");
Debug.Log("The lowest number you can pick is: " + minNumToGuess + ".");
Debug.Log("Tell me if your number is higher or lower than 500.");
Debug.Log("Press UP ARROW if higher, Press DOWN ARROW if lower, Press ENTER if correct.");
maxNumToGuess += 1;
}
void Update ()
{
if (Input.GetKeyDown(KeyCode.UpArrow))
{
Debug.Log("You told me your number was higher...");
minNumToGuess = guess;
guess = NewGuess();
}
else if (Input.GetKeyDown(KeyCode.DownArrow))
{
Debug.Log("You told me your number was lower...");
maxNumToGuess = guess;
guess = NewGuess();
}
else if (Input.GetKeyDown(KeyCode.KeypadEnter) || Input.GetKeyDown(KeyCode.Return))
{
Debug.Log("Hey look I guessed your number. WIZARD!");
Debug.Log("Your number was: " + guess + ".");
}
}
public int NewGuess()
{
int newGuess = (maxNumToGuess + minNumToGuess) / 2;
Debug.Log("Let me guess again ... what about: " + newGuess + "?");
return newGuess;
}
}
/* Known bug, if the user incorrectly gives the correct input for their guess, the guess will eventually get stuck on a single number when the minimum guess and maximum guess become equal to one another. */
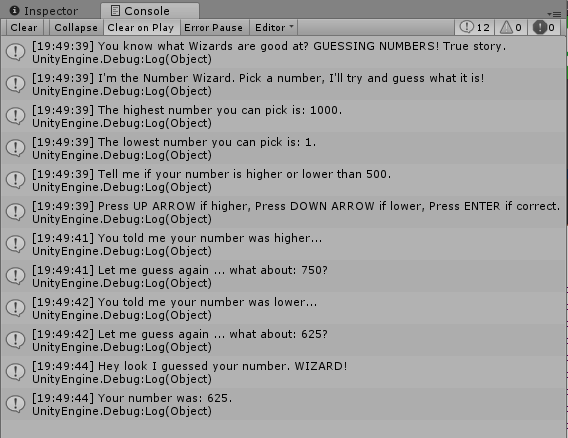