There are no One Size Fits All solutions to this issue, unfortunately. Raycasting corner of each gridPosition would catch the issue found in the original post, but would miss a Cylindrical object that never actually touches the corner.
Placing colliders on the tiles themselves (placing them on the visual perhaps?) could work, though you have to be careful that the colliders don’t also intersect with the floor, and this could complicate things on a multi-floor or a map with simple elevations.
I use a trick that actually works well for both square and hex tiles. I use a script that can be searched for by the Pathfinding
public class PathfindingObstacle : MonoBehaviour
{
public GridPosition GetPosition()=>LevelGrid.Instance.GetGridPosition(transform.position);
}
This simply acts as a tag. Then Pathfinding can generate it’s list of obstacles by simply searching for them.
foreach (var obstacle in FindObjectsByType<PathfindingObstacle>(FindObjectsSortMode.None))
{
GridPosition position = obstacle.GetGridPosition();
GetNode(position.x, position.z).SetIsWalkable(false);
}
This still leaves us a problem, however. This will work fantastically well for obstacles that are single units, but it doesn’t work quite so well for a wall of multiple units. For this, we have to get a bit creative…
Let’s take a look at the OP’s drawing:
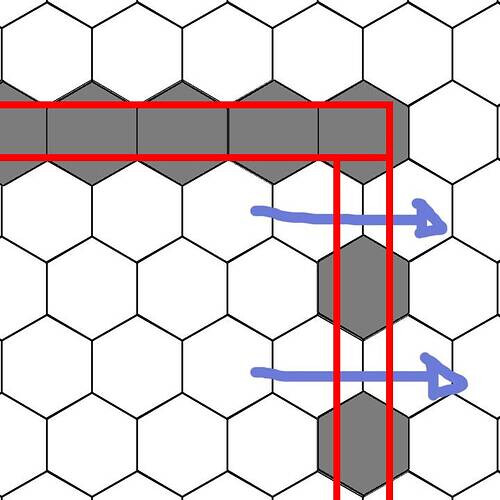
In this scenario, I"m actually going to assume that each of these walls is the same prefab, copied once horizontally and once vertically. What we need are child GameObjects at each potential GridPosition. So what I did was add pairs of GameObjects with PathFindingObstacles spaced left and right of the lengthwise center of the wall, just enough to be into the adjacent gridpositions if they overlap the bar, and close enough in distance from the other pairs to ensure that every GridPosition is covered.
Consider these two walls (each using the same prefab)…
For this next image, I turned the renderer off to show the locations of the PathfindingObstacles
The outlines are the Box Colliders, which as the OP reported don’t reach the centers when the wall North/South. As you can see these colliders are spaced so that they are each within a hex tile.
Now here’s the best part… if two or more PathfindingObstacles are in the same GridPosition, it doesn’t matter. We’re only using these to mark a position as unwalkable. Multiple entries will just keep calling the tile unwalkable.