I am getting this NullReferenceException… again. The first time I was able to solve it because I compared my code with Gary’s side by side and I was able to find out I had two lines at the start that Gary was not using… but this one I don’t know what’s wrong.
Annoyingly it keeps sending me to the if statement from the DisplayAnswer(), but I am not sure what’s wrong there… I even copied and pasted Gary’s code, and it prompted the same Exception.
In the Inspector it seems alright, the list is there and the questions are there, the timer the scoring and the sprites are there, the onClick even is also populated and the scripts also there, so I am not sure what’s wrong
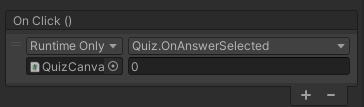

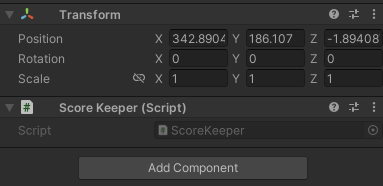
This is my own code following Gary’s videos:
`using System.Collections;
using System.Collections.Generic;
using TMPro;
using UnityEngine;
using UnityEngine.UI;
public class Quiz : MonoBehaviour
{
[Header("Questions")]
[SerializeField] TextMeshProUGUI questionText;
[SerializeField] List<QuestionSO> questions; //= new List<QuestionSO>();
QuestionSO currentQuestion;
[Header("Answers")]
[SerializeField] GameObject[] answerButtons;
int correctAnswerIndex;
bool hasAnsweredEarly;
int index;
[Header("Button Colors")]
[SerializeField] Sprite defaultSprite;
[SerializeField] Sprite correctAnswerSprite;
[Header("Timer")]
[SerializeField] Image timerImage;
Timer timer;
[Header("Scoring")]
[SerializeField] TextMeshProUGUI scoreText;
ScoreKeeper scoreKeeper;
void Start()
{
//DisplayAnswer(index);
timer = FindObjectOfType<Timer>();
//DisplayQuestion();
scoreKeeper = FindObjectOfType<ScoreKeeper>();
}
private void Update()
{
timerImage.fillAmount = timer.fillFraction;
if(timer.loadNextQuestion)
{
hasAnsweredEarly = false;
GetNextQuestion();
timer.loadNextQuestion = false;
}
else if(!hasAnsweredEarly && !timer.isAnsweringQuestion)
{
DisplayAnswer(-1);
SetButtonState(false);
}
}
public void OnAnswerSelected(int index)
{
hasAnsweredEarly = true;
DisplayAnswer(index);
SetButtonState(false);
timer.CancelTimer();
scoreText.text = "Score: " + scoreKeeper.CalculateScore() + "%";
}
void DisplayAnswer(int index)
{
Image buttonImage;
if (index == currentQuestion.GetCorrectAnswerIndex())
{
questionText.text = "Correct!";
buttonImage = answerButtons[index].GetComponent<Image>();
buttonImage.sprite = correctAnswerSprite;
scoreKeeper.IncrementCorrectAnswers();
}
else
{
correctAnswerIndex = currentQuestion.getCorrectAnswerIndex();
string correctAnswer = currentQuestion.getCorrectAnswer(correctAnswerIndex);
questionText.text = "Sorry, the correct answer was "; //+ correctAnswer;
buttonImage = answerButtons[correctAnswerIndex].GetComponent<Image>();
buttonImage.sprite = correctAnswerSprite;
}
}
void GetNextQuestion()
{
if(questions.Count > 0)
{
SetButtonState(true);
SetDefaultButtonSprites();
GetRandomQuestion();
DisplayQuestion();
scoreKeeper.IncrementQuestionsSeen();
}
}
void GetRandomQuestion()
{
index = Random.Range(0,questions.Count);
currentQuestion = questions[index];
if(questions.Contains(currentQuestion))
{
questions.Remove(currentQuestion);
}
}
void DisplayQuestion()
{
questionText.text = currentQuestion.GetQuestion();
for (int i = 0; i < answerButtons.Length; i++)
{
TextMeshProUGUI buttonText = answerButtons[i].GetComponentInChildren<TextMeshProUGUI>();
buttonText.text = currentQuestion.getCorrectAnswer(i);
}
}
void SetButtonState(bool state)
{
for(int i = 0;i < answerButtons.Length; i++)
{
Button button = answerButtons[i].GetComponent<Button>();
button.interactable = state;
}
}
void SetDefaultButtonSprites()
{
for(int i = 0; i < answerButtons.Length; i++)
{
Image buttonSprite = answerButtons[i].GetComponent<Image>();
buttonSprite.sprite = defaultSprite;
}
}
}
`