What does main do:
main function helps to give a start point to the computer that this is the most important block to look into
What does const do:
const helps to maintain the variable as a constant
No assignment possible
tried to do it and error was:
cannot assign a variable to that is constant
#include preprocessor directives
these are used to include a copy of all the codes in the header files for a ready to use case
int main()
main function is the start point for the function to look into
expression statements
statements are the lines ending with ;
expression statements are the ones which either output or assign a value
declaration statements
these are statements where the variables are declared
Return statement
this is a statement that is executed at the end of the code and its execution means the code is successfully run
// preprocessor directives
#include <iostream>
//Intro
void PlayIntro(int LevelDifficulty)
{
//expression statements
//c = 25;assigning before initializing causes error undeclared indentifier
std::cout<<"\nzoldar is collapsing!! please input code to get your pod!!! " << "\n";
std::cout<<"the level difficulty is now at "<< LevelDifficulty<<" crack to break and live!!!!"<<"\n";
//endl ends and line and prints a new one
std::cout<<"There are many levels and u need to enter the correct code to continue...\n";
std::cout<<"Enter three inputs seperated by spaces\n";
}
//Game play
bool PlayGame(int LevelDifficulty)
{
//Function call
PlayIntro(LevelDifficulty);
//declaration statements
const int CodeA = 4;
const int CodeB = 2;
const int CodeC = 4;
//intialize a variable and declare
int PlayersGuessA, PlayersGuessB, PlayersGuessC ;
//Input statements
std::cin>>PlayersGuessA>>PlayersGuessB>>PlayersGuessC;
//calculation statements(declaration statements)
const int CodeSum = CodeA + CodeB + CodeC;
const int CodeProd = CodeA * CodeB * CodeC;
const int GuessSum = PlayersGuessA + PlayersGuessB + PlayersGuessC;
const int GuessProduct = PlayersGuessA * PlayersGuessB * PlayersGuessC;
//character output << insertion opeartor
std::cout<<"the sum of the numbers is " << CodeSum;
std::cout<< std::endl;
std::cout<<"the product is "<<CodeProd <<std::endl;
std::cout<<"The sum for the given numbers is given is "<<GuessSum<<"\n";
std::cout<<"The product for the given numbers is given as "<<GuessProduct<<"\n";
//win lose conditon
if(CodeSum == GuessSum)
{
std::cout<<"The initial barrier for level 1 is now open\n";
if(CodeProd == GuessProduct){
std::cout<<"Yes you are one step closer to save our species from extension\n";
return true;
}
else{
std::cout<<"You failed zloadar's life!!\n";
return false;
}
}
else
{
std::cout<<"You failed zloadar's life!!\n";
return false;
}
}
//Main function
int main()
{
int LevelDifficulty = 1;
const int MaxLevelDifficulty = 10;
while(LevelDifficulty <= MaxLevelDifficulty)
{
//boolean and initialize
bool bLevelComplete = PlayGame(LevelDifficulty);
//functioncall
std::cin.clear();
std::cin.ignore();
if(bLevelComplete)
{
LevelDifficulty = LevelDifficulty + 1;
}
else
{
LevelDifficulty = LevelDifficulty;
}
}
//return statement
return 0;
}
Unreal screen namings
This is the main view port
modes areas where we can find all the assets and gameplay objects
Main tool bar where most of the functionalities like save and build and compile are found
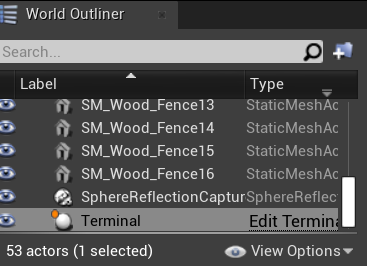
World outliner, an higher up view of all the things going on in the editor
Details tab where we can find the complete details of all the assets in one place
Go to edit-> editor preferences → Play and game gets mouse control to have full mouse control during the game
Define actors:
Any asset in the game or the program can be considered as an actor it may be a light, a prop the main character etc all are actors
They all have a transform
What is a transform?? They all have coordinates they adhere to and they also have rotation and scale properties
Use surface snapping options to snap the object or the actor to a specific surface
select the surface snapping option as on and use the origin of the gimble to snap the object to the surface nearby
End button makes the actor to stick back on the ground(nearest surface)
Helps the object rotate to the normal to the floor keeps the object perpendicular at all points to the ground normal/on or off as per requirements(Rotate to surface normal)
Tips:
U want to locate the world center, use location 0,0,0 for the global center
The coordinates are all global, so the 0,0,0 point remains the main point to iterate the distances
Use the copy paste options for the transform so that it will be easy to duplicate things
Note!!!
Multiple select in unreal is shift not control!!!
lock is a special option for scale where each scale property is translated equally after lock
example: if 2 1 1 is the scale when locked then when 2 is updated to 4 the scale now becomes 4 2 2
the same holds good for 1 1 1 as well, if the scale is locked at 1 1 1 then once the scale of one axis is updated the rest auto updates
Use alt key or cntrl+c to copy and duplicate meshes
gimble is the only way to move the meshes no keyboard shortcuts
F is used to focus on the asset selected