Hi guys!
I think I followed the lesson correctly but Unity is giving me an error when its time to destroy the enemy prefab:
I’m using Unity 2020.1.17f1
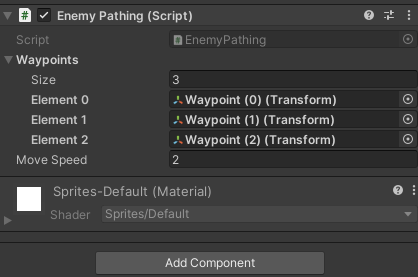
public class EnemyPathing : MonoBehaviour
{
[SerializeField] List<Transform> waypoints;
[SerializeField] float moveSpeed = 2f;
int waypointIndex = 0;
private void Start()
{
transform.position = waypoints[waypointIndex].transform.position;
}
private void Update()
{
Move();
}
private void Move()
{
var targetPosition = waypoints[waypointIndex].transform.position;
var movementThisFrame = moveSpeed * Time.deltaTime;
if (waypointIndex <= waypoints.Count - 1)
{
transform.position = Vector2.MoveTowards(transform.position, targetPosition, movementThisFrame);
if (transform.position == targetPosition)
{
waypointIndex++;
}
}
else
{
Destroy(gameObject);
}
}
}