Or instead of getting the object through the tag grab the reference directly. Add a [SerializeField] private Transform cameraTransform; Then in the editor drag that Main camera object you have selected and remove the cameraTransform = Camera.main.transform;
I can’t figure how to attach it to a prefab. I added it to CameraController and made CameraController a singleton. Had each LookAt use that camera, still no improvement.
You must have some other object also using the MainCamera tag. Did you do Debug.Log(cameraTransform); and it said “Main Camera (UnityEngine.Transform)”? If so then search in the hierarchy for another object named “Main Camera”, you should find 2
I added a debug log to Testing to list all game objects with MainCamera:
private void Start()
{
var items = GameObject.FindGameObjectsWithTag("MainCamera");
Debug.LogWarning("Found items: " + items.Length.ToString());
}
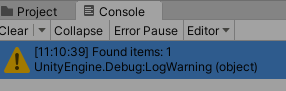
I’m thinking something else affecting must be the key, or something so simple I’m completely missing it.
Here’s the camera controller:
public class CameraController : MonoBehaviour
{
public static CameraController Instance;
private const float MIN_FOLLOW_Y_OFFSET = 2.0f;
private const float MAX_FOLLOW_Y_OFFSET = 24.0f;
public Camera mainCamera;
[SerializeField] private CinemachineVirtualCamera cinemachineVirtualCamera;
private CinemachineTransposer transposer;
private Vector3 targetFollowOffset;
[SerializeField] private float moveSpeed = 8.0f;
[SerializeField] private float rotationSpeed = 100.0f;
[SerializeField] private float zoomSpeed = 5.0f;
private void Awake() {
if (Instance == null) Instance = this;
}
private void Start() {
transposer = cinemachineVirtualCamera.GetCinemachineComponent<CinemachineTransposer>();
targetFollowOffset = transposer.m_FollowOffset;
}
// Update is called once per frame
void Update() {
HandleMovement();
HandleRotation();
HandleZoom();
}
private void HandleMovement() {
Vector3 inputMoveDirection = Vector3.zero;
if (Input.GetKey(KeyCode.W)) {
inputMoveDirection.z = +1.0f;
}
if (Input.GetKey(KeyCode.S)) {
inputMoveDirection.z = -1.0f;
}
if (Input.GetKey(KeyCode.A)) {
inputMoveDirection.x = -1.0f;
}
if (Input.GetKey(KeyCode.D)) {
inputMoveDirection.x = +1.0f;
}
inputMoveDirection.Normalize();
Vector3 moveVector = transform.forward * inputMoveDirection.z + transform.right * inputMoveDirection.x;
transform.position += moveVector * moveSpeed * Time.deltaTime;
}
private void HandleRotation() {
Vector3 rotationVector = Vector3.zero;
if (Input.GetKey(KeyCode.Q)) {
rotationVector.y = +1.0f;
}
if (Input.GetKey(KeyCode.E)) {
rotationVector.y = -1.0f;
}
transform.eulerAngles += rotationVector * rotationSpeed * Time.deltaTime;
}
private void HandleZoom() {
targetFollowOffset.y = targetFollowOffset.y - Input.mouseScrollDelta.y;
targetFollowOffset.y = Mathf.Clamp(targetFollowOffset.y, MIN_FOLLOW_Y_OFFSET, MAX_FOLLOW_Y_OFFSET);
transposer.m_FollowOffset = Vector3.Lerp(transposer.m_FollowOffset, targetFollowOffset, zoomSpeed * Time.deltaTime);
}
}