I’m having a bizzare issue with the score display singleton in Laser Defender.
When I start my game from the start scene or play again from the game over
scene, the score does not update as I kill enemies but it is being calculated
correctly because when I die, I get the correct final score in the game over
scene. However, when I start my game from the game scene, the score DOES
update properly but does not carry over to the game over scene, the final
score is always 0. I’m not getting any errors. I have ScoreDisplay.cs attached
to a Score Text within Game Canvas in both my Game and Game Over scenes and
GameSession.cs attached to a Game Session object in both scenes as well. Any
idea what is happening here?
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ScoreDisplay : MonoBehaviour
{
Text scoreText;
GameSession gameSession;
// Start is called before the first frame update
void Start()
{
scoreText = GetComponent<Text>();
gameSession = FindObjectOfType<GameSession>();
}
// Update is called once per frame
void Update()
{
scoreText.text = gameSession.GetScore().ToString();
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GameSession : MonoBehaviour
{
int score = 0;
private void Awake()
{
SetUpSingleton();
}
private void SetUpSingleton()
{
int numberGameSessions = FindObjectsOfType<GameSession>().Length;
if(numberGameSessions > 1)
{
Destroy(gameObject);
}
else
{
DontDestroyOnLoad(gameObject);
}
}
public int GetScore()
{
return score;
}
public void AddToScore(int scoreValue)
{
score += scoreValue;
}
public void ResetGame()
{
Destroy(gameObject);
}
}
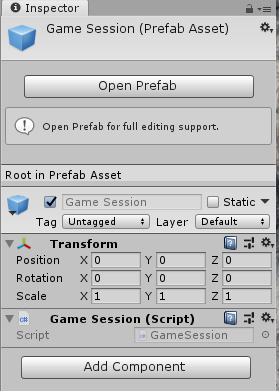