Added the debugs in the PlayerAttackingState script, it looks like it should work, it’s calling TryApplyForce after the normalizedTime is greater than ForceTime, however the “dash foward” seems to wait until the animation reaches the Combo Attack Time value.
I don’t know if it has something to do with the animations:
I tested all the animations with an without the “Root Transform …” boxes ticked, in both the problem persists, again only for attack 2 and 3.
And here’s how the animator is set up:
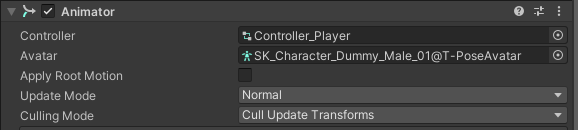
Here’s the log from a complete sequence (I removed some of the similar logs so it would fit the character limit, there’s a description of what happens in bold):
normalizedTime = 0, attack.ForceTime = 0.35
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0.00320711, attack.ForceTime = 0.35
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
(normalizedTime is going up)
normalizedTime = 0.3478479, attack.ForceTime = 0.35
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0.3500842, attack.ForceTime = 0.35
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime > attack.ForceTime, calling TryApplyforce(), alreadyAppliedForce == False
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:40)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0.3523012, attack.ForceTime = 0.35
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime > attack.ForceTime, calling TryApplyforce(), alreadyAppliedForce == True
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:40)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0.3550049, attack.ForceTime = 0.35
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime > attack.ForceTime, calling TryApplyforce(), alreadyAppliedForce == True
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:40)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
(normalizedTime is going up)
normalizedTime = 0.5005897, attack.ForceTime = 0.35
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime > attack.ForceTime, calling TryApplyforce(), alreadyAppliedForce == True
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:40)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0, attack.ForceTime = 0.45
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0.002291874, attack.ForceTime = 0.45
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
(normalizedTime is going up)
normalizedTime = 0.4492868, attack.ForceTime = 0.45
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0.4509192, attack.ForceTime = 0.45
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime > attack.ForceTime, calling TryApplyforce(), alreadyAppliedForce == False
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:40)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0.4524879, attack.ForceTime = 0.45
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime > attack.ForceTime, calling TryApplyforce(), alreadyAppliedForce == True
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:40)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0.4542135, attack.ForceTime = 0.45
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime > attack.ForceTime, calling TryApplyforce(), alreadyAppliedForce == True
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:40)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
(normalizedTime is going up)
normalizedTime = 0.600233, attack.ForceTime = 0.45
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime > attack.ForceTime, calling TryApplyforce(), alreadyAppliedForce == True
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:40)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0, attack.ForceTime = 0.8
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0.00135268, attack.ForceTime = 0.8
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
(normalizedTime is going up)
normalizedTime = 0.7973536, attack.ForceTime = 0.8
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0.8003838, attack.ForceTime = 0.8
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime > attack.ForceTime, calling TryApplyforce(), alreadyAppliedForce == False
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:40)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0.8017328, attack.ForceTime = 0.8
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime > attack.ForceTime, calling TryApplyforce(), alreadyAppliedForce == True
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:40)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime = 0.8049088, attack.ForceTime = 0.8
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime > attack.ForceTime, calling TryApplyforce(), alreadyAppliedForce == True
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:40)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
(normalizedTime is going up)
normalizedTime = 0.9986878, attack.ForceTime = 0.8
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:37)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)
normalizedTime > attack.ForceTime, calling TryApplyforce(), alreadyAppliedForce == True
UnityEngine.Debug:Log (object)
PlayerAttackingState:Tick (single) (at Assets/Scripts/StateMachines/Player/PlayerAttackingState.cs:40)
StateMachine:Update () (at Assets/Scripts/StateMachines/StateMachine.cs:19)