The really weird part is that the compiler thinks Barrel is an FVector…
The very first error is:
expression must have pointer type
then
error C2819: type ‘FVector’ does not have an overloaded member ‘operator ->’
ok - header for TankAimingComponent (.h)
#pragma once
#include "Components/ActorComponent.h"
#include "TankAimingComponent.generated.h"
UCLASS( ClassGroup=(Custom), meta=(BlueprintSpawnableComponent) )
class BATTLETANK_API UTankAimingComponent : public UActorComponent
{
GENERATED_BODY()
public:
// Sets default values for this component's properties
UTankAimingComponent();
void AimAt(FVector HitLocation);
void SetBarrelReference(UStaticMeshComponent* BarrelToSet);
protected:
// Called when the game starts
virtual void BeginPlay() override;
public:
// Called every frame
virtual void TickComponent(float DeltaTime, ELevelTick TickType,
FActorComponentTickFunction* ThisTickFunction) override;
private:
UStaticMeshComponent* Barrel = nullptr;
};
and now the CPP file:
// Fill out your copyright notice in the Description page of Project Settings.
#include "BattleTank.h"
#include "TankAimingComponent.h"
// Sets default values for this component's properties
UTankAimingComponent::UTankAimingComponent()
{
// Set this component to be initialized when the game starts, and to be ticked every frame. You can turn these features
// off to improve performance if you don't need them.
PrimaryComponentTick.bCanEverTick = true;
// ...
}
void UTankAimingComponent::SetBarrelReference(UStaticMeshComponent * BarrelToSet)
{
Barrel = BarrelToSet;
}
// Called when the game starts
void UTankAimingComponent::BeginPlay()
{
Super::BeginPlay();
// ...
}
// Called every frame
void UTankAimingComponent::TickComponent(float DeltaTime, ELevelTick TickType,
FActorComponentTickFunction* ThisTickFunction)
{
Super::TickComponent(DeltaTime, TickType, ThisTickFunction);
// ...
}
void UTankAimingComponent::AimAt(FVector HitLocation)
{
auto OurTankName = GetOwner()->GetName();
auto BarrelLocation = Barrel->GetComponentLocation()->ToString();
UE_LOG(LogTemp, Warning, TEXT("Tank %s aiming at: %s from %s"), *OurTankName,
*HitLocation.ToString(), *BarrelLocation);
}
Now look, even intellisense “knows” it’s a pointer… !!!
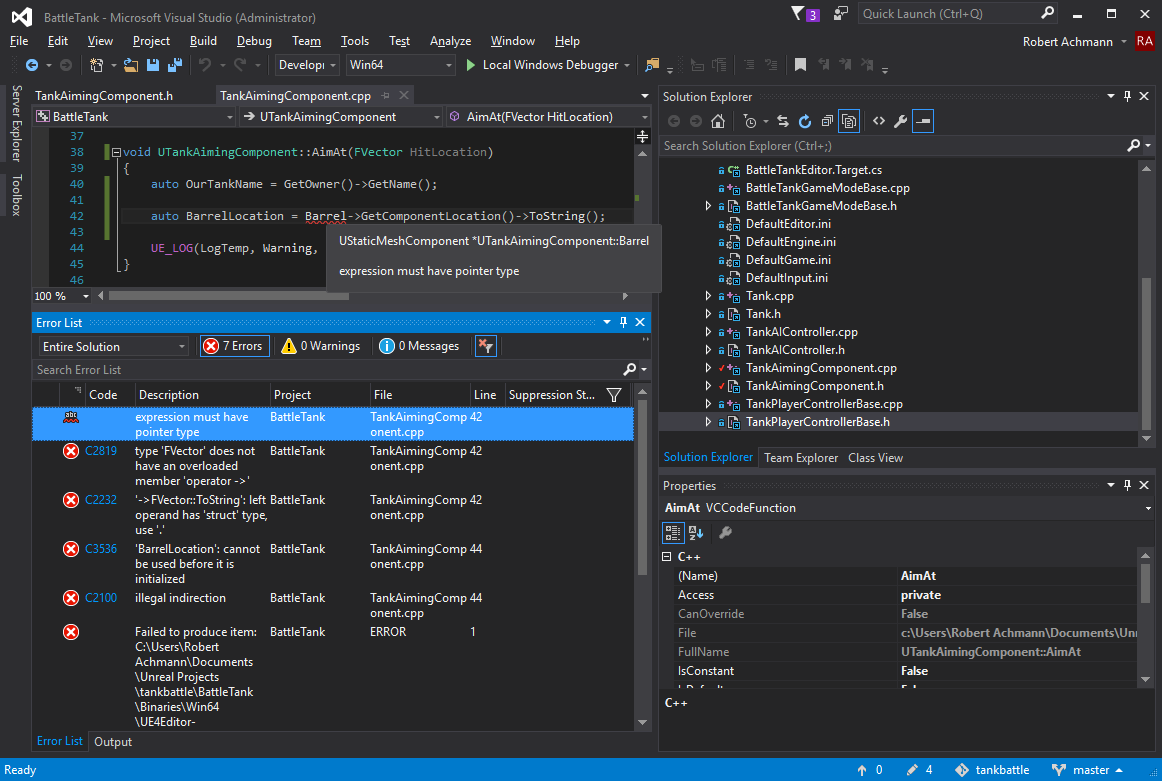