There are several ways to achieve this but all comes down to vector math and rotations.
Consider that each object has two type of scales and rotations; global and local, this is very important because based on this you can give all sorts of directions to a missile or even calculate if the player is in front of an enemy.
Let’s say you have an object, then, you write this block of code to a script and attach it to that object:
void Update()
{
transform.Translate(Vector2.right, Space.World);
}
The object will move to its right regardless of rotation, but what if you change the line to this: transform.Translate(Vector2.right, Space.Self);
, the object will move completely different based on its rotation, the difference is that the first uses a global vector, while the second uses the local vector. You can even see the difference in the Editor window if you press the Global buttton at the right of the selection tools!
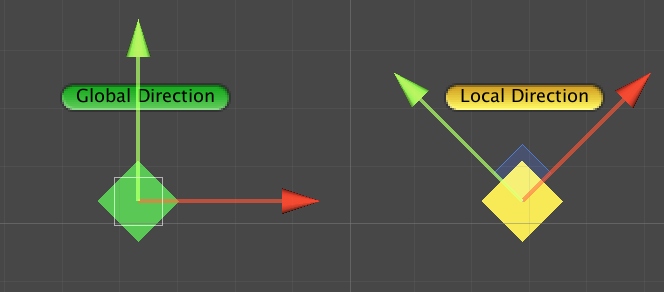
In the image below, the triangles on the left are using a global direction while the ones on the right are using a local direction, it changes quite a lot.
This way you can pass down the rotation of something to a moving object. That’s usually how Twin Stick games work (Enter The Gungeon, The Ascent), you have a character that rotates and when it shoots, it pases down his local direction to the missiles making them move to where the character is aiming.
There are many other ways to achieve that, like subtracting vectors to get the direction between the two.
It’s better if you test things out by yourself, so why not copy-paste the code below and have fun with it. Attach this to any 2D object and play with the exposed variables and the Z rotation.
[SerializeField] float speed = 0.1f;
[SerializeField] bool useGlobalDirection = true;
void Update()
{
Space spaceType = useGlobalDirection ? Space.World : Space.Self;
transform.Translate(Vector2.right * speed * Time.deltaTime, spaceType);
}
Keep in mind that you won’t always have acces to the Space
type, in those cases to get the local direction of an object you can use the transform
type; transform.right
, transform.forward
, or transform.up
will always return you the local direction of the transform.